IndentationError: expected an indented block
The "IndentationError: expected an indented block" in Python occurs when a code block that requires indentation is missing or not correctly indented. Python uses indentation to define the scope of control structures like loops, conditionals, and function definitions. If the expected indentation is not provided after a statement that requires a block, such as "if," "for," or "def," Python raises this error. Let's explore some examples and how to resolve them:
Missing Indented Block
In this example, an "if" statement is used, but there is no indented block following it. Python expects an indented block after the "if" statement to define the code to execute if the condition is True. Since the block is missing, Python raises the "IndentationError: expected an indented block."
To fix this, add the required indentation to define the block inside the "if" statement:
Incorrect Indentation Level
In this example, the "for" loop is missing the proper indentation. Python requires an indented block after the "for" statement to define the loop body. Without the correct indentation, Python raises the "IndentationError: expected an indented block."
To fix this, ensure the loop body is indented correctly:
Here are some tips for avoiding indentation errors:
- Use a consistent indentation style.
- Use a code editor that has a built-in indent checker.
- Use the autopep8 or black tools to automatically format your code.
Python block
In Python, after a colon (:) appears, the subsequent line-break and indented block define a code block. Python relies on white-space, which can be spaces or tabs, to distinguish and delineate code blocks. When multiple statements share the same indentation level, they are considered part of the same block. Python strictly requires consistent indentation within a block for separate statements. Therefore, it will raise an error both when an indentation is missing where a block is expected and when varying indentations are used within a block, enforcing adherence to a standardized indentation style. By maintaining a uniform and consistent indentation practice, Python developers can ensure code readability, maintainability, and prevent potential errors due to indentation inconsistencies.
Indentation in Python
Indentation in Python can be achieved using any consistent whitespace, but adhering to a recommended standard is crucial for maintaining clean and readable code. It is generally advised to utilize 4 spaces for indentation in Python, as it strikes a balance between accommodating deeper nesting of code blocks and enhancing code readability. While tabulation or a different number of spaces may be feasible, they can occasionally lead to complications. Opting for spaces exclusively is often the preferable approach. To facilitate consistent indentation, most editors offer an option to automatically convert tabs to spaces.
Activating this feature in your editor can promote adherence to the recommended indentation style, ensuring uniformity throughout your codebase and facilitating seamless collaboration among developers. By embracing standard indentation practices, Python developers can maintain code consistency and readability, optimizing code maintainability and minimizing the likelihood of errors stemming from inconsistent indentation.
Tabbed indentation
Using tabs for indentation in Python code can lead to inconsistencies due to varying spacing interpretations across different editors. Moreover, mixing tabbed indentation with spaced indentation can be confusing and undermine code clarity. To ensure a standardized indentation approach, it is advisable to use spaces exclusively. If you are working with an Integrated Development Environment (IDE) such as Eclipse, you have the flexibility to configure the number of spaces the IDE inserts when you press the tab key.
While modern IDEs typically assist in maintaining indentation, it remains essential to exercise caution and properly indent code blocks to maintain code readability and prevent errors. Since whitespace characters might not be visible in certain editors, developers must be vigilant in applying consistent indentation to facilitate code collaboration and minimize potential discrepancies. By adhering to a consistent and space-based indentation practice, Python developers can create code that is easier to read, maintain, and understand.
Docstring Indentation
The "IndentationError: expected an indented block" error can also occur when a programmer forgets to indent a docstring. Docstrings must follow the indentation rules of the surrounding code in a function. When docstring processing tools are used, they will remove a consistent amount of indentation from the second and subsequent lines of the docstring, which is equivalent to the minimum indentation found among all non-blank lines after the first line.
To avoid this error, it is essential to ensure that docstrings are indented correctly and consistently with the surrounding code. By adhering to proper indentation practices, docstrings can be effectively processed by tools without raising an "IndentationError," allowing for clear and accurate documentation of Python functions. This attention to detail helps maintain code readability and ensures that docstrings contribute effectively to the overall documentation of the codebase.
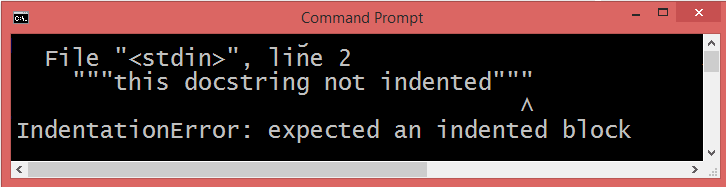
To fix this issue, indent the docstring .
Conclusion
The "IndentationError: expected an indented block" in Python can be resolved by providing the necessary indentation after control statements like "if," "for," and "def" to define the associated code blocks correctly. Consistent and appropriate indentation is crucial for maintaining proper code structure and ensuring the correct execution of Python programs.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- ValueError: invalid literal for int() with base 10
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'