SyntaxError- EOL while scanning string literal
An EOL ( End of Line ) error indicates that the Python interpreter expected a particular character or set of characters to have occurred in a specific line of code, but that those characters were not found before the end of the line . This results in Python stopping the program execution and throwing a syntax error .

The SyntaxError: EOL while scanning string literal error in python occurs when while scanning a string of a program the python hit the end of the line due to the following reasons:
- Missing quotes
- Strings spanning multiple lines
Missing quotes
output
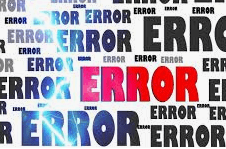
The reason for this error is that forgot a closing double quote at the end of the string. String literals can be enclosed in matching single quotes (') or double quotes ("). They can also be enclosed in matching groups of three single or double quotes (these are generally referred to as triple-quoted strings).
output
Strings spanning multiple lines
output
The reason for this error is that a string enclosed in single or double quotes can't span multiple lines . Strings can't normally span multiple lines. A multiline string in Python begins and ends with either three single quotes (''') or three double quotes ("""). Any quotes, tabs, or newlines in between the "triple quotes" are considered part of the string. Python's indentation rules for blocks do not apply to lines inside a multiline string .
output
Python is particularly prone to this type of error, since Python ends statements with newlines/line breaks , whereas most other programming languages have a character such as a semicolon (;) , which means that other programming languages work more easily with multi-line statements out of the box.

Syntax errors
Syntax errors are produced by Python when it is translating the source code into byte code. They usually indicate that there is something wrong with the syntax of the program. Syntax errors are usually easy to fix once you figure out what they are. Unfortunately, the error messages are often not helpful. A common cause of syntax errors is the difference in syntax between Python 2 and Python 3. In particular, a syntax error may be alerted if a Python 3 file is assumed to be compatible with Python 2 (or vice versa). Explicitly specifying the expected Python version can help prevent this.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- ValueError: invalid literal for int() with base 10
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'