invalid literal for int() with base 10
The error message "invalid literal for int() with base 10" suggests that you are attempting to use the int() function with a string that does not represent a valid integer. This error occurs when the string is either empty or contains characters other than digits, making it unsuitable for direct conversion to an integer. To address this issue, you must ensure that the string being passed to the int() function contains only valid numeric characters in base 10 representation.
By validating the input string and handling cases where it does not conform to the requirements for integer conversion, you can prevent the occurrence of the "invalid literal for int() with base 10" error and ensure the smooth execution of your code. Adopting a proactive approach to handle potential invalid inputs enhances the robustness and reliability of your Python program.
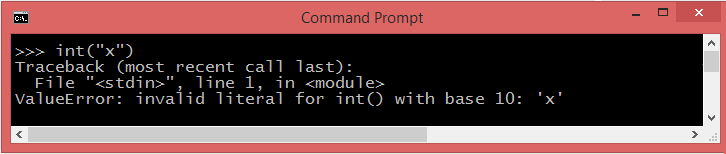
int() method
The int() method in Python is a built-in function designed to convert a given number or string into an integer data type. By default, the conversion is performed in base 10. When provided with a valid number or string, this method returns an integer object that corresponds to the converted value. In cases where no arguments are provided to the method, it returns 0 as the default value. By utilizing the int() function appropriately, developers can effortlessly transform numeric values or strings into integer representations, facilitating seamless numerical operations and enhancing the efficiency and readability of Python code.

But you get a ValueError: invalid literal for int() with base 10 , if you pass a string representation of a float into int , or a string representation of anything but an integer (including empty string).
How to fix it?
Python isdigit()
To resolve this error, you can employ Python's isdigit() method to verify whether the value is a number or not. This method returns True if all the characters in the value are digits, indicating that it is a valid number. Otherwise, it returns False, signifying that the value contains non-numeric characters. By using the isdigit() method to perform this validation, you can ensure the proper handling of input values and prevent errors related to non-numeric data.
This approach maintains robustness in your Python code, as it allows for accurate determination of the nature of the input and enables appropriate actions based on the result of the isdigit() evaluation. Applying this method ensures smooth execution and enhances the reliability of your Python programs when dealing with numeric data.
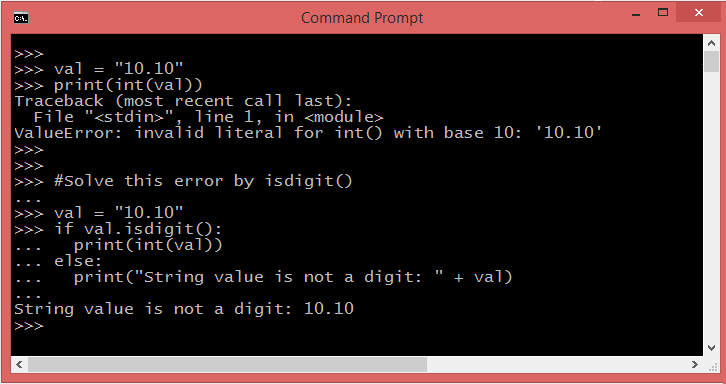
Using try-except
Another effective approach to address this issue is to encapsulate your code within a Python try...except block, providing a robust way to handle potential errors. By utilizing this error-handling mechanism, you can anticipate and manage exceptions that might occur during the execution of your code, including situations where the int() method encounters an error due to invalid input. When an exception is raised, the code inside the except block is executed, allowing you to implement appropriate error-handling procedures, provide informative error messages, or take corrective actions as needed.
By incorporating try...except blocks, you fortify your Python code with a resilient error-handling mechanism, promoting stability and appropriate degradation even in the face of unexpected situations. Embracing this professional practice not only enhances the reliability of your code but also contributes to a more robust and user-friendly Python application.
Floating-Point Numbers
When attempting to convert a floating-point string (e.g., "10.10") to an integer in Python, it is essential to apply a two-step conversion process for accurate results. First, invoke the float() function to transform the float string into a floating-point number representation. Then, subsequently, apply the int() function to the floating-point number to achieve the desired integer conversion. This approach ensures precise handling of the float string and enables a seamless transition from a string containing a floating-point value to an integer representation. By following this professional technique, Python developers can effectively manage data type conversions and maintain the integrity and accuracy of their numeric data, enhancing code reliability and robustness.
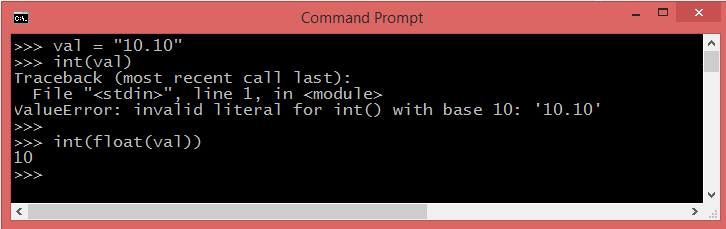
The above code converts the string ("10.10") to a floating point value, which is then converted to an integer via truncation—that is, by discarding the fractional part . Applying these functions to "10.10" will produce a result of 10. If, on the other hand, you wanted the floating point value , just use only float().
Python2.x and Python3.x
The occurrence of the ValueError: invalid literal for int() with base 10 can be attributed to the disparity between Python 2.x and Python 3.x in their handling of certain numeric operations. In Python 2.x, using int(str(3/2)) results in the integer "1," while in Python 3.x, the same operation yields the float value "1.5," leading to the ValueError.
The discrepancy stems from Python 3.x's stricter type-checking and adherence to strong typing principles. In such scenarios, it is crucial to be mindful of these version-specific differences to ensure proper data type conversions and prevent errors during the execution of Python code. By embracing version-aware coding practices and appropriately handling numeric operations, developers can ensure compatibility and smooth execution across different Python versions, developing code robustness and enhancing user experience.
Python excels at abstracting low-level details from developers, making it effortless to work with numerical data. While most other programming languages also support double precision floating-point numbers, Python's design philosophy ensures that you need not concern yourself with such intricacies. Since version 3.0, Python has introduced automatic conversion of integers to floats when performing division, simplifying numeric computations significantly. This feature enhances the ease of working with numerical values in Python and reduces the need for explicit type conversions. By using these built-in capabilities, Python developers can focus on high-level problem-solving and enjoy the simplicity and efficiency that the language offers.
Computers use different representations to store numbers, and Python offers two primary ones: Integers, which hold whole numbers without any fractional part, and floating-point numbers, which store real numbers with decimal fractions. "Base 10" refers to the decimal numbering system, where we count using digits from 0 to 9. Choosing the appropriate data type is essential, depending on the nature of the numbers you are working with. Integers are suitable for whole numbers, while floating-point numbers are ideal for handling real numbers that may have decimal parts. By using the appropriate data type based on the specific requirements of your program, you can ensure accurate representation and computation of numbers, optimizing performance and precision in your Python code.
Conclusion
To resolve the "invalid literal for int() with base 10" error, ensure that the string being passed to the int() function contains only valid numeric characters in base 10 representation. Handling invalid characters or formatting appropriately will allow you to convert the string to an integer successfully.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'