TypeError: string indices must be integers
An Iterable is a collection of elements that can be accessed sequentially . In Python, iterable objects are indexed using numbers . When you try to access an iterable object using a string or a float as the index, an error will be returned.
All the characters of a string have a unique index . This index specifies the position of each character of the string. TypeError: string indices must be integers means an attempt to access a location within a string using an index that is not an integer. In the above case using the str[hello"] and str[2.1] as indexes. As these are not integers, a TypeError exception is raised. This means that when you're accessing an iterable object like a string or float value, you must do it using an integer value .
In the above example, instead of providing str[hello"] or str[2.1] to the string, provided an integer value str[4]. Thus no error is encountered. Since string indices only accept integer value.

Dictionary example
When you run the above code, you will get the output like:
Here, TypeError: string indices must be integers has been caused because you are trying to access values from dictionary using string indices instead of integer.
If you are accessing items from a dictionary , make sure that you are accessing the dictionary itself and not a key in the dictionary. The value of "i" is always a key in the dictionary . It's not a record in the dictionary. Let's try to using "i" in the dictionary example:
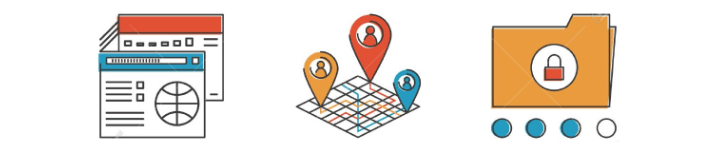
Slice Notation string[x:y]
Python supports slice notation for any sequential data type like lists, strings , tuples, bytes, bytearrays, and ranges. When working with strings and slice notation, it can happen that a TypeError: string indices must be integers is raised, pointing out that the indices must be integers, even if they obviously are.
A comma "," is actually enough for Python to evaluate something as a tuple. So here you need to replace the comma "," with a colon ":" to separate the two integers correctly.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- ValueError: invalid literal for int() with base 10
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'