How to fix IndexError: string index out of range
Strings are an essential part of just about any programming language. A string is an array of characters . The string index out of range means that the index you are trying to access does not exist. In a string, that means you're trying to get a character from the string at a given point. If that given point does not exist , then you will be trying to get a character that is not inside of the string.

example
output
Let's take the above example:
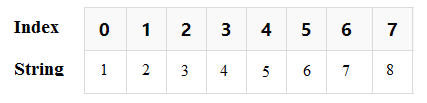
try following code:
But what happens if we request index 14?
output
Here we get a string index out of range , because we are asking for something that doesn't exist. In Python, a string is a single-dimensional array of characters. Indexes in Python programming start at 0. This means that the maximum index for any string will always be length-1 . Here that makes your numbers[8] fail because the requested index is bigger than the length of the string.

The string index out of range problem has to do with a very common beginner problem when accessing elements of a string using its index. There are several ways to account for this. Knowing the length of your string could certainly help you to avoid going over the index.
When you run len() function on "numbers" you get the length of our string as 8. Just note that the length doesn't start at 0, it starts at 1. Since Python uses zero-based indexing , the maximum index of a string is the length of the string minus one. So, you can access the maximum index value of a string is its length minus one .
Handling errors and exceptions is another topic in itself, but here briefly show how to prevent it with string indices.
output
In the above example, the error handled it carefully .
- TypeError: 'NoneType' object is not subscriptable
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- ValueError: invalid literal for int() with base 10
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'