Uncaught ReferenceError: $ is not defined
jQuery is a JavaScript library designed to simplify and enhance JavaScript programming. It indeed aims to make coding tasks more efficient and user-friendly. While working with jQuery, encountering JavaScript errors is a common occurrence, but jQuery provides various methods and techniques to handle and resolve these errors effectively.
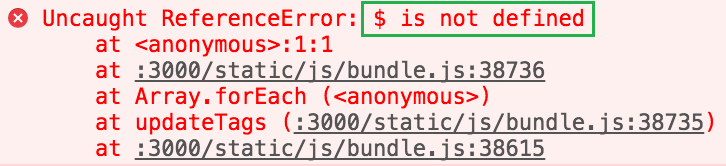
The symbol "$" serves as an alias for the jQuery() function. Consequently, attempting to invoke or access it prior to its formal declaration will invariably result in an error message stating that "$" is undefined. This commonly signals an issue with jQuery's absence or JavaScript's inability to recognize the "$" symbol. Even when employing "$(document).ready", the "$" symbol remains undefined, as jQuery has not been loaded at that point in the execution process.
To solve this error:
To ensure that the "$" symbol or "jQuery" is recognized in your scripts, it is advisable to load the jQuery library at the outset of all your JavaScript files. This practice enables the proper identification and utilization of "$" or "jQuery" throughout your scripts, ensuring seamless functionality.
exampleIn the following code, you can see that the jQuery library is loaded after the script. So, you will get "$ is not defined" error.
In order to fix this error, include the jQuery library file before the JavaScript code.

Click Here...
There can be multiple other reasons for this issue:
Working offline

In situations where you may find yourself working offline and encountering difficulties loading jQuery from an internet-based Content Delivery Network (CDN) due to connectivity issues, a prudent approach is to download the jQuery.js file and utilize it locally. To achieve this, you can employ the following code snippet to load jQuery from a local source:
By specifying the correct path to your locally stored jQuery.js file within the src attribute of the <script> tag, you can ensure that jQuery is loaded and utilized locally, mitigating potential connectivity problems associated with CDN-based loading.
Conflict with Other Libraries
jQuery employs the "$" sign as a convenient shorthand, but conflicts can arise when using other JavaScript libraries such as Angular, Backbone, prototype.js, MooTools, and others that also utilize the "$" variable. To circumvent such conflicts and maintain compatibility, you can employ the noConflict() method. This method allows you to continue using jQuery by referencing it with its full name, "jQuery," rather than the "$" shortcut, ensuring smooth coexistence with other libraries:
Path to jQuery library you included is not correct
You might have put an incorrect path to jQuery library , or there may be typo in your file.
The above code does not include the HTTP: or HTTPS: in the src attribute but the browser, FireFox, needed it so you should changed it to:
The jQuery library file is corrupted
If the jQuery library file has been downloaded from an untrustworthy sites and the file is corrupted, this error may happen.

Google CDN:
Microsoft CDN
Conclusion
The error message "Uncaught ReferenceError: $ is not defined" indicates that the symbol "$" is being used in JavaScript code without prior definition, usually occurring when jQuery is not properly loaded or conflicts with other libraries using the same symbol. To resolve this error, ensure jQuery is loaded correctly or consider using the noConflict() method to prevent conflicts with other libraries.
- jQuery Interview Questions (Part-2)
- jQuery Interview Questions (Part-3)
- Is jQuery a programming language?
- Why do we need to go for JQuery?
- How to check jQuery version?
- How to multiple version of jQuery?
- What is jQuery CDN?
- Advantages of minified version of JQuery
- How do I check if the DOM is ready?
- How to Use the jQuery load() Method
- Difference between document.ready() and body onload()?
- Is jQuery is a replacement of JavaScript?
- JQuery or JavaScript which is quicker in execution?
- What is the use of param() method in jquery
- How to work with jQuery parent(), children() and siblings()?
- Difference between parent() and parents() in jQuery?
- What does jQuery data() function do?
- How do you check if an element exists or not in jQuery?
- How do I check if an HTML element is empty using jQuery?
- How to run an event handler only once in jQuery?
- How to Disable or Enable a Form Element Using jQuery
- Hide and show image on button click using jQuery
- Difference Between Prop and Attr in jQuery
- How do I check if an element is hidden in jQuery?
- Difference between return false; and e.preventDefault()
- What is each() function in jQuery? How do you use it?
- Which one is more efficient, document.getElementbyId( "myId") or $("#myId)?
- What is the difference between $.map and $.grep in jQuery
- What is the use of serialize method in jQuery
- What is the use of clone method in jQuery?
- What is event.PreventDefault in jQuery?
- Difference between event.PreventDefault and event.stopPropagation?
- What are deferred and promise object in jQuery?
- What are source maps in jQuery?
- What does the jQuery migrate function do?
- Differences Between jQuery .bind() and .live()?
- How can you delay document.ready until a variable is set?
- How to disable cut,copy and paste in TextBox using jQuery?
- How to prevent Right Click option using jquery?
- How does the jQuery pushStack function work?
- Why use jQuery filter() Methods?
- Difference between find() and closest() in jquery?
- How To Use Ajax In Jquery?
- How to multiple AJAX requests be run simultaneously in jQuery?
- Can we call C# code behind using jQuery?
- How to include jQuery in ASP.Net project?
- Need to add jQuery file in both Master and Content page?
- Uncaught TypeError: $(…).modal is not a function jquery
- How to check whether a checkbox is checked in jQuery?
- How to Convert JSON Date to JavaScript/jQuery date