jQuery Interview Questions (Part-2)
Is jQuery a W3C standard?
No, jQuery is not a W3C standard. It is a popular JavaScript library developed by the jQuery team to simplify client-side scripting.
Is jQuery a client or server scripting?
jQuery is a client-side scripting library. It runs in the web browser of the client (user's device) and is used for enhancing the user interface and interactivity of web pages.
Can jQuery Run on MAC or Linux instead of Windows?
Yes, jQuery is platform-independent and can run on MAC, Linux, or Windows operating systems as long as the web browser being used supports JavaScript.
Where is jQuery code getting executed?
jQuery code is executed within the web browser of the client, which means it runs on the client's machine.
Which is the starting point of code execution in jQuery?
The starting point of code execution in jQuery is often the $(document).ready() function, which ensures that the code runs after the DOM (Document Object Model) is fully loaded and ready to be manipulated.
What are all the ways to include jQuery in a page?
You can include jQuery in a page by downloading it and referencing it using a local path or by using a Content Delivery Network (CDN) link. Common CDNs include Google CDN and jQuery's official CDN.
Can we use a protocol-less URL while referencing jQuery from CDNs?
Yes, you can use a protocol-less URL (starting with //) when referencing jQuery from CDNs. This allows the browser to automatically use the appropriate protocol (HTTP or HTTPS) based on the page's current protocol.
How to resolve conflicts with other libraries?
To resolve conflicts with other libraries using the $ (dollar sign) symbol, you can use jQuery's noConflict() method to release control of the $ variable and use a different alias. For example: jQuery.noConflict();.
How to execute jQuery code after the DOM is ready?
You can execute jQuery code after the DOM is ready by using $(document).ready(function() { /* your code here */ }); or the shorthand $(function() { /* your code here */ });.
Which sign is used as a shortcut for jQuery?
The dollar sign $ is commonly used as a shortcut for jQuery. For example, $(selector) is equivalent to jQuery(selector).
Can you use any other name in place of $ (dollar sign) in jQuery?
Yes, you can use any valid JavaScript variable name in place of $ by using the jQuery.noConflict() method. This allows you to avoid conflicts with other libraries that may also use $.
Can we add more than one "document.ready" function on a page?
Yes, you can add multiple $(document).ready() functions on a page, and they will all execute when the DOM is ready. This is useful for organizing your code into separate blocks.
What is the difference between "#" and "." selector in jQuery?
# is used to select elements by their id attribute, while . is used to select elements by their class attribute in jQuery selectors. For example, $("#myId") selects an element with the id of "myId," and $(".myClass") selects elements with the class "myClass."
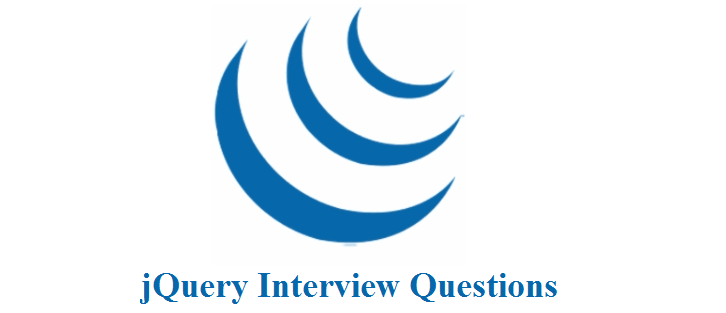
How can we write code specific to a browser in jQuery?
You can write browser-specific code in jQuery by using conditional statements to check the browser's user agent string and then execute different code based on the browser type and version.
What is the difference between jQuery and jQuery UI?
jQuery is a library for DOM manipulation and AJAX interactions, while jQuery UI is a separate library built on top of jQuery that focuses on user interface components and interactions, such as widgets and effects.
Difference between remove() and empty()?
remove() removes the selected element(s) from the DOM, including all its descendants and data. empty() removes the contents (child elements and text) of the selected element(s) but leaves the element itself in place.
What is has() in jQuery?
has() is a jQuery method used to filter the set of matched elements to include only those that have a specific descendant element. It helps you select elements based on the presence of other elements within them.
- jQuery Interview Questions (Part-3)
- Is jQuery a programming language?
- Why do we need to go for JQuery?
- How to check jQuery version?
- How to multiple version of jQuery?
- What is jQuery CDN?
- Advantages of minified version of JQuery
- How do I check if the DOM is ready?
- How to Use the jQuery load() Method
- Difference between document.ready() and body onload()?
- Is jQuery is a replacement of JavaScript?
- JQuery or JavaScript which is quicker in execution?
- What is the use of param() method in jquery
- How to work with jQuery parent(), children() and siblings()?
- Difference between parent() and parents() in jQuery?
- What does jQuery data() function do?
- How do you check if an element exists or not in jQuery?
- How do I check if an HTML element is empty using jQuery?
- How to run an event handler only once in jQuery?
- How to Disable or Enable a Form Element Using jQuery
- Hide and show image on button click using jQuery
- Difference Between Prop and Attr in jQuery
- How do I check if an element is hidden in jQuery?
- Difference between return false; and e.preventDefault()
- What is each() function in jQuery? How do you use it?
- Which one is more efficient, document.getElementbyId( "myId") or $("#myId)?
- What is the difference between $.map and $.grep in jQuery
- What is the use of serialize method in jQuery
- What is the use of clone method in jQuery?
- What is event.PreventDefault in jQuery?
- Difference between event.PreventDefault and event.stopPropagation?
- What are deferred and promise object in jQuery?
- What are source maps in jQuery?
- What does the jQuery migrate function do?
- Differences Between jQuery .bind() and .live()?
- How can you delay document.ready until a variable is set?
- How to disable cut,copy and paste in TextBox using jQuery?
- How to prevent Right Click option using jquery?
- How does the jQuery pushStack function work?
- Why use jQuery filter() Methods?
- Difference between find() and closest() in jquery?
- How To Use Ajax In Jquery?
- How to multiple AJAX requests be run simultaneously in jQuery?
- Can we call C# code behind using jQuery?
- How to include jQuery in ASP.Net project?
- Need to add jQuery file in both Master and Content page?
- Uncaught TypeError: $(…).modal is not a function jquery
- How to check whether a checkbox is checked in jQuery?
- Uncaught ReferenceError: $ is not defined
- How to Convert JSON Date to JavaScript/jQuery date