jQuery Effects: Hide/Show Image
jQuery hide() and show()
In jQuery, you can use the .hide() and .show() methods to control the visibility of HTML elements on a webpage.
- The .hide() method is used to hide elements by setting their CSS display property to "none," effectively making them invisible.
- The .show() method is used to display hidden elements, reverting their CSS display property to its original state.
These methods are commonly used for creating interactive and dynamic web pages, allowing you to control when and how elements are displayed to users.

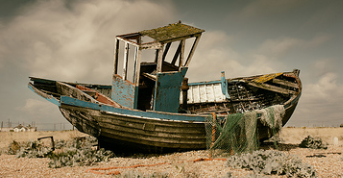
jQuery toggle() method
The jQuery toggle() method is used to toggle between the hide() and show() methods for the selected elements. It provides a simple way to toggle the visibility of elements on a web page.
Here's a brief explanation of how it works:
- When you call toggle(), it checks the current visibility state of the selected element(s).
- If the element(s) is currently visible (displayed), it hides it using the hide() method.
- If the element(s) is currently hidden (not displayed), it shows it using the show() method.

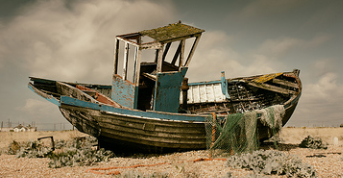
Note:
The .toggle() method had its event-based signature deprecated in jQuery 1.8 and subsequently removed in jQuery 1.9. However, jQuery still offers an animation-oriented .toggle() method that facilitates the toggling of element visibility, incorporating smooth transitions like sliding elements up or down. The specific behavior of this method—whether it functions as an animation or event handler—is determined by the arguments provided during its invocation, making it a versatile tool for creating interactive and visually appealing elements in web development.
Conclusion
jQuery provides the .hide() and .show() methods, which allow you to easily hide and show HTML elements, including images, on a webpage. These methods are commonly used to create interactive effects and control element visibility in web development.
- jQuery Interview Questions (Part-2)
- jQuery Interview Questions (Part-3)
- Is jQuery a programming language?
- Why do we need to go for JQuery?
- How to check jQuery version?
- How to multiple version of jQuery?
- What is jQuery CDN?
- Advantages of minified version of JQuery
- How do I check if the DOM is ready?
- How to Use the jQuery load() Method
- Difference between document.ready() and body onload()?
- Is jQuery is a replacement of JavaScript?
- JQuery or JavaScript which is quicker in execution?
- What is the use of param() method in jquery
- How to work with jQuery parent(), children() and siblings()?
- Difference between parent() and parents() in jQuery?
- What does jQuery data() function do?
- How do you check if an element exists or not in jQuery?
- How do I check if an HTML element is empty using jQuery?
- How to run an event handler only once in jQuery?
- How to Disable or Enable a Form Element Using jQuery
- Difference Between Prop and Attr in jQuery
- How do I check if an element is hidden in jQuery?
- Difference between return false; and e.preventDefault()
- What is each() function in jQuery? How do you use it?
- Which one is more efficient, document.getElementbyId( "myId") or $("#myId)?
- What is the difference between $.map and $.grep in jQuery
- What is the use of serialize method in jQuery
- What is the use of clone method in jQuery?
- What is event.PreventDefault in jQuery?
- Difference between event.PreventDefault and event.stopPropagation?
- What are deferred and promise object in jQuery?
- What are source maps in jQuery?
- What does the jQuery migrate function do?
- Differences Between jQuery .bind() and .live()?
- How can you delay document.ready until a variable is set?
- How to disable cut,copy and paste in TextBox using jQuery?
- How to prevent Right Click option using jquery?
- How does the jQuery pushStack function work?
- Why use jQuery filter() Methods?
- Difference between find() and closest() in jquery?
- How To Use Ajax In Jquery?
- How to multiple AJAX requests be run simultaneously in jQuery?
- Can we call C# code behind using jQuery?
- How to include jQuery in ASP.Net project?
- Need to add jQuery file in both Master and Content page?
- Uncaught TypeError: $(…).modal is not a function jquery
- How to check whether a checkbox is checked in jQuery?
- Uncaught ReferenceError: $ is not defined
- How to Convert JSON Date to JavaScript/jQuery date