Unexpected EOF while parsing
Unexpected EOF( End Of File ) while parsing is a syntax error which means the end of source code is reached even before all the blocks of code are completed. This happens in a number of situations in Python, such as:
- Missing or unmatched parentheses.
- Forget to enclose code inside a special statement.
- Unfinished try statement.
Missing or unmatched parentheses
This can simply also mean you are missing a closing parenthesis or curly bracket in a block of code or have too many parentheses.
The above statement will result in unexpected EOF because the statement misses the closing parentheses ")". You can correct it by add the closing parentheses.
In order to avoid the above situations, you should keep your code clean and concise, reducing the number of operations occurring on one line where suitable.
Forget to enclose code inside a special statement
In this case, Python can't find an indented block of code to pair with your statement.
In the above statement, a code block starts with a statement like for i in range(50): and requires at least one line afterwards that contains code that should be in it. So, the above statement will result in unexpected EOF because the statement forget to enclose code inside for loop statement.
To avoid this error, you have to enter the whole code block as a single input:
Unfinished try statement
This is another situation that produced this exception: if you write a try block without any except or finally will result in unexpected EOF.
In the above statement, a try block without any except or finally clause. So this will result in unexpected EOF because Python is expecting at least one except or finally clause.
To avoid this error, you should write except or finally clause with your try statement.
Or
What is EOF in Python?
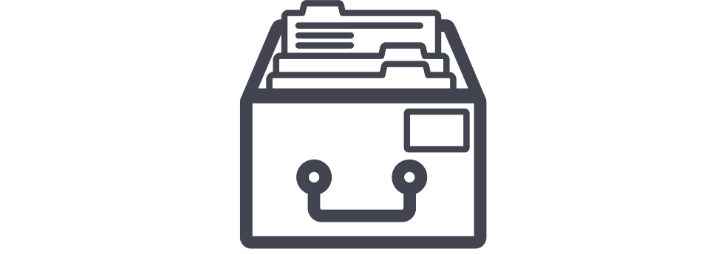
EOF stands for "End Of File", is a special delimiter or data that is placed at the end of a file after the last byte of data in the file. This represents the last character in a Python program.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- ValueError: invalid literal for int() with base 10
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'