IndexError: list index out of range
In Python, lists which are mutable that means the size is not fixed. So, you can add items to these lists after that have been already declared and you can access its elements by its index . You can add as many items as you want, but now the question arises; what happens if you try to access the item which is not in the list ?

This error basically means you are trying to access a value at a List index which is out of bounds i.e greater than the last index of the list or less than the least index in the list. So the first element is 0, second is 1, so on. So if there are n elements in a list, the last element is n-1 . If you try to access the empty or None element by pointing available index of the list, then you will get the "List index out of range " error. To solve this error, you should make sure that you're not trying to access a non-existent item in a list.
Python IndexError problem has to do with a very common beginner problem when access List elements by its index.
output
Here you can see, the IndexError is raised when attempting to retrieve an index from a sequence, and the index isn't found in the sequence.
Length of list is 3, so if you access elements in list whose index greater than 2 then it will show an error:
Here index is 3 which is equal to length of list so you can't access it. If you have a list with 3 items, the last one is MyList[2] because the way Python indexing works is that it starts at 0.
The IndexError: "Index Out of Range" can occur for all ordered collections where you can use indexing to retrieve certain elements.
Indexing
An index in Python refers to a position within an ordered list . To retrieve an element of the list, you use the index operator ([]) . Using indexing you can easily get any element by its position. Python strings can be thought of as lists of characters ; each character is given an index from zero (at the beginning) to the length minus one (at the end).
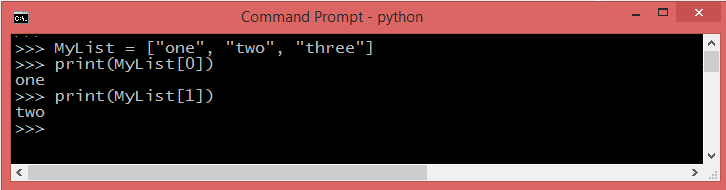
Lists are "zero indexed" , so MyList[0] returns the left-most (i.e. zero-th) item in the list, and MyList[1] returns the second item (i.e. one item to the right of the zero-th item). Since there are 3 elements in your list (MyList[0] through MyList[2]), attempting to access MyList[3] throws an IndexError: list index out of range , since it is actually trying to get the fourth element, and there isn't one.
You can access the List elements at the end by adding a minus also.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- ValueError: invalid literal for int() with base 10
- AttributeError: 'module' object has no attribute 'main'
- UnboundLocalError: local variable referenced before assignment
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'