Button Control in ASP.NET
The Button control in ASP.NET is designed to render a push button element on a Web page. By default, a Button control acts as a Submit button, triggering form submission when clicked. It provides a visually identifiable and interactive element for users to initiate actions or trigger specific operations on the server.
Button control
To define the behavior of a Button control, developers can provide an event handler for the Click event. This event is fired when the button is clicked by the user. By wiring up the Click event handler, developers can programmatically control the actions performed when the button is clicked, allowing for custom logic and server-side processing.
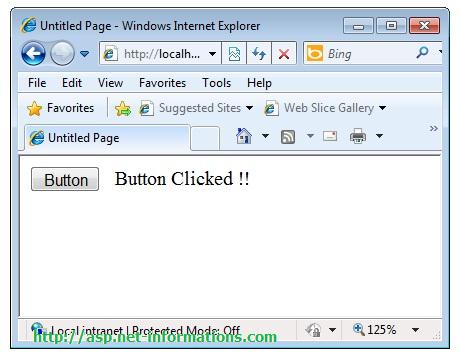
Within the event handler for the Click event, developers can write code to handle the button click event. This code can include various operations, such as validating user input, processing data, interacting with a database, or triggering other server-side actions. The event handler provides a convenient way to encapsulate the desired functionality associated with the button click.
VB.NetCustomizing the event handler for the Click event, developers have the flexibility to control the specific actions and behavior when the button is clicked. This empowers them to create dynamic and interactive experiences, respond to user input, and perform server-side operations based on the click event.
The following ASP.NET program display a text in the label when the Button click event is performed.
Default.aspx
Conclusion
The Button control in ASP.NET enables the display of a push button element on a Web page. Its default behavior is that of a Submit button, but developers can customize the actions performed by providing an event handler for the Click event. This allows for programmatically controlling the behavior and actions associated with the button when it is clicked by the user.