Textbox Control in Asp.Net
In Asp.Net, the TextBox server control serves as a versatile input control, designed to accept user input within web applications. By default, TextBoxes are configured to accommodate a single line of text, providing a simple yet effective means for users to provide their input. However, it is important to note that the flexibility of the TextBox control extends beyond its default behavior, enabling developers to enhance user experience by utilizing advanced features.
Multiline text input
One notable capability of the TextBox control is its ability to support multiline text input. By altering the value of the TextMode property to TextBoxMode.MultiLine, developers can effortlessly transform a TextBox into a more expansive and accommodating text box, capable of accepting multiple lines of input. This empowers users to express themselves more comprehensively, particularly in scenarios where longer textual content or structured input is required.
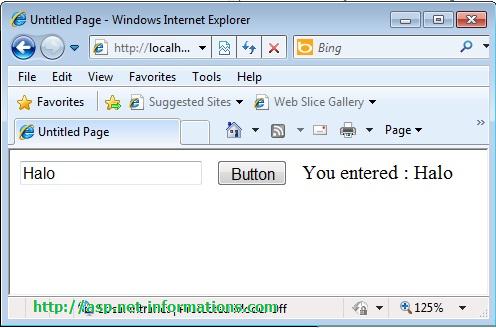
Another valuable feature of the TextBox control is its capacity to mask user input. By modifying the TextMode property to TextBoxMode.Password, developers can use the TextBox control to create password fields, ensuring that sensitive information remains hidden from prying eyes. This essential functionality enhances security and confidentiality, safeguarding user credentials and other sensitive data.
VB.NetIf you want to limit the user input to a specified number of characters, set the MaxLength property.
VB.NetThe following ASP.NET program retrieve user input from a TextBox control and display it to a Label control.
Defaul.aspx