Checkbox Control in ASP.NET
CheckBoxes in ASP.NET provide a convenient way for users to make multiple selections from a list of options. Each CheckBox is associated with a caption or label, which can be set using the Text property of the CheckBox control.
Checkbox Control
The CheckBox control represents a binary state, allowing users to either select or deselect an option by clicking on the checkbox. When a CheckBox is selected, it displays a checkmark indicating its state. Multiple CheckBox controls can be placed on a web page, each representing a different option.
Developers can set the caption or label for a CheckBox by assigning the desired text to the Text property. This caption provides descriptive information about the option being represented by the CheckBox control, making it easier for users to understand the purpose of each checkbox.
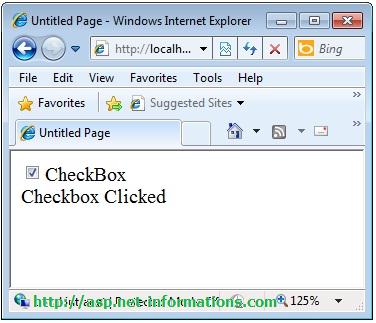
The state of CheckBox controls can be programmatically accessed and manipulated in server-side code. Developers can handle the CheckedChanged event of the CheckBox control to perform actions or trigger specific behaviors based on the checkbox selection.
Checkbox is selected (clicked) or not
The checked property of a CheckBox control allows you to determine whether the checkbox is selected (clicked) or not. The checked property is a boolean value that indicates the state of the CheckBox control.
By accessing the checked property, you can programmatically determine whether the checkbox is checked or unchecked. This property can be used in server-side code to perform specific actions or make decisions based on the checkbox's state.
VB.NetThe state of CheckBox controls can be programmatically accessed and manipulated in server-side code. Developers can handle the CheckedChanged event of the CheckBox control to perform actions or trigger specific behaviors based on the checkbox selection.
CheckBox controls are commonly used in scenarios where users need to make multiple selections from a list of options, such as selecting multiple items for a shopping cart, choosing preferences, or indicating membership to multiple groups or categories.
The following ASP.Net program detect whether the Checkbox is clicked or not and display the message on Label control.
Default.aspxConclusion
CheckBoxes in ASP.NET provide a straightforward and intuitive mechanism for enabling users to make multiple selections. The accompanying caption, set through the Text property, enhances usability by providing clear descriptions of the options associated with each CheckBox control.