Calendar control in ASP.NET
The Calendar control in ASP.NET is a useful tool for presenting a calendar view on a web page. It enables users to select dates and navigate through different months. The control provides several features to customize its appearance and behavior, allowing developers to tailor it to their specific needs.
To utilize the Calendar control, you can include it in your ASP.NET markup (e.g., in an .aspx file) using the <asp:Calendar> tag.
In this example, the Calendar control with the ID "calendarExample" is added to the web page.
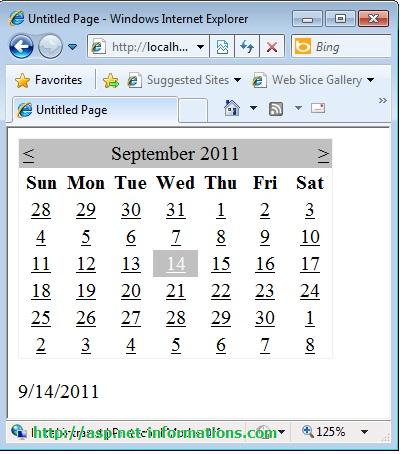
Calendar control properties
The Calendar control provides various properties that enable you to customize its appearance. For instance, you can modify the style of different elements within the control, such as the header, days, and selected date, by setting the appropriate properties.
In this example, the HeaderStyle-BackColor property is set to "Red" to change the background color of the calendar's header, the DayStyle-ForeColor property is set to "Blue" to change the text color of the days, and the SelectedDayStyle-Font-Bold property is set to "true" to make the selected date's font bold.
Additionally, the Calendar control offers functionality for handling events such as date selection or month navigation. You can handle the SelectionChanged event to perform specific actions when a date is selected by the user.
In the server-side code, you can define the corresponding event handler method:
This event handler allows you to execute custom logic when the user selects a date in the Calendar control.
The following ASP.NET program display the selected Calender date in short date format in a label control.
Default.aspxConclusion
The Calendar control in ASP.NET provides an efficient way to display a calendar on a web page. It offers customization options through properties that control the style of different elements. Additionally, it supports events for handling user interactions, enabling developers to create dynamic and interactive calendar experiences for their web applications.