Label Control in ASP.NET
The Label control in ASP.NET serves the purpose of displaying text at a specific location on a Web page. It provides a convenient means to present static or dynamically generated content to the user. The displayed text can be easily customized using the Text property of the Label control.
Label control
With the Label control, developers can define a fixed position on the page where the text will be rendered. This allows for precise placement and alignment within the overall page layout. The Label control can be positioned using HTML and CSS techniques, enabling seamless integration with other elements and styling.
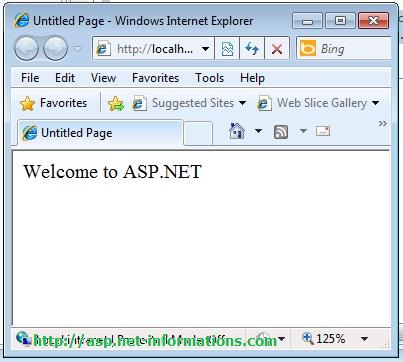
The content displayed by the Label control can be static, hardcoded text set directly in the Text property, or it can be dynamically generated based on data or other runtime conditions. This flexibility allows developers to create dynamic and interactive user interfaces that respond to user actions or present real-time information.
C#To customize the displayed text, developers can easily modify the Text property of the Label control programmatically. This allows for dynamic updates to the displayed content, such as changing text based on user input, retrieving data from a database, or incorporating logic to generate specific messages or instructions.
The following ASP.NET program display a text in a Label control through its Text property.
Default.aspxConclusion
The Label control is a versatile tool in ASP.NET that simplifies the display of text in a set location on a Web page. Its Text property provides an accessible means to customize the displayed content, making it an essential component for creating informative and visually appealing user interfaces.