DropDownList Control in ASP.NET
The DropDownList control in ASP.NET is designed to provide a dropdown menu that allows users to select a single item from a list of options. It offers a user-friendly interface for presenting a set of choices and enables users to make a selection by clicking on the dropdown arrow and choosing an item from the list.
DropDownList control
The DropDownList control can accommodate any number of items, allowing developers to populate it with a dynamic or static list of options. The items can be defined in the markup of the control or programmatically added during runtime, based on data retrieved from a database, web service, or other sources.
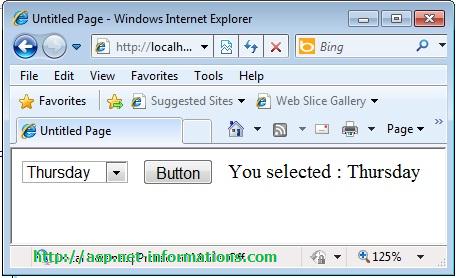
When the user interacts with the DropDownList, they can click on the dropdown arrow, which expands the list of available options. The user can then scroll through the options and select a single item by clicking on it. The selected item is displayed in the dropdown area when the list collapses, providing visual feedback to the user.
A default ListItem can be added to a DropDownList programmatically with the following syntax after binding data to the DropDownList:
VB.NetDevelopers can also capture the user's selection by handling the SelectedIndexChanged event of the DropDownList control. This event is triggered when the selected item changes, allowing developers to perform specific actions or implement logic based on the user's selection.
The DropDownList control is widely used in ASP.NET for scenarios where users need to make a single selection from a predefined set of options. It is commonly used for selecting a category, choosing a state or country, picking a product from a list, or any other situation where a dropdown menu with a single selection is required.
The following ASP.NET program add seven days in a week to the DropDownList in the form load event and display the selected item in the button click event.
Default.aspx
Conclusion
The DropDownList control enhances the user experience by providing an intuitive and convenient way to choose a single item from a list of options. Its versatility and ease of use make it a valuable component in ASP.NET web applications.