Image Control in ASP.NET
The ASP.NET Image control is a powerful tool for displaying images on a web page in an ASP.NET application. It provides various features and properties to customize the appearance and behavior of the displayed images.
Image control
To use the Image control, you need to add it to your ASP.NET markup (e.g., in an .aspx file) and specify the source of the image using the ImageUrl property. Here's an example:
In this example, the Image control with the ID "imgExample" is set to display the image located at the "~/Images/example.jpg" path.
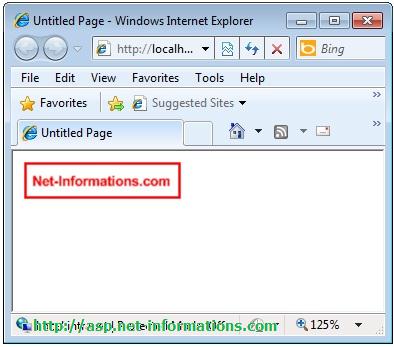
AlternateText property
The Image control also supports alternative text through the AlternateText property, which provides a textual description of the image for accessibility purposes. This text is displayed when the image fails to load or when the user is utilizing assistive technologies. Here's an example:
VB.NetDynamic modification
In addition to the basic properties, the Image control allows for dynamic modification of its properties through server-side code. For example, you can change the ImageUrl property programmatically based on certain conditions. Here's an example in C#:
This code demonstrates how you can change the ImageUrl property of the Image control dynamically based on the value of a condition.
The Image control supports various events such as Click, MouseOver, and MouseOut, allowing you to perform actions or trigger server-side code when the user interacts with the image. Here's an example:
In this example, the OnClick event is wired up to the "imgExample_Click" method in the server-side code. When the user clicks on the image, the specified method is executed.
The following ASP.NET program load an Image from a URL and set an alternate text.
Default.aspx
Conclusion
The ASP.NET Image control offers a convenient and flexible way to display images on web pages in an ASP.NET application. It provides various properties and events to control the image's appearance, behavior, and interactivity, making it a valuable component for creating visually engaging and interactive web applications.