Listbox Control in ASP.NET
The ListBox in ASP.NET control provides a means to display a list of selectable items on a web page. There are several methods and properties available for adding items and customizing the behavior of the ListBox control:
Adding Items
- The Add method is used to append items to the end of an unsorted ListBox control.
- The Insert method allows you to specify the position where the item should be inserted within the ListBox.
ListBox1.Items.Add("Sunday")
C#
ListBox1.Items.Add("Sunday");
Selection Mode
- The ListBox control supports both single and multiple item selection modes.
- To enable multiple item selection, you can set the SelectionMode property to ListSelectionMode.Multiple. This allows users to select multiple items simultaneously by holding down the Ctrl or Shift key.
ListBox1.SelectionMode = ListSelectionMode.Multiple
C#
ListBox1.SelectionMode = System.Web.UI.WebControls.ListSelectionMode.Multiple;
The following ASP.NET program add seven days in a week to the ListBox in the load event and display the selected item in the label.
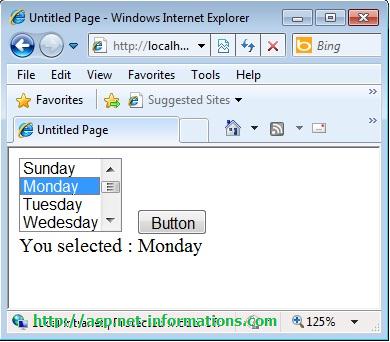
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ListBox ID="ListBox1" runat="server"></asp:ListBox>
<asp:Button ID="Button1" runat="server" Text="Button" onclick="Button1_Click" />
<br />
<asp:Label ID="Label1" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
Full Source | C#
using System;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
ListBox1.Items.Add("Sunday");
ListBox1.Items.Add("Monday");
ListBox1.Items.Add("Tuesday");
ListBox1.Items.Add("Wednesday");
ListBox1.Items.Add("Thursday");
ListBox1.Items.Add("Friday");
ListBox1.Items.Add("Saturday");
}
protected void Button1_Click(object sender, EventArgs e)
{
Label1.Text = "You selected : " + ListBox1.SelectedItem.ToString();
}
}
Full Source | VB.NET
Partial Class _Default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
ListBox1.Items.Add("Sunday")
ListBox1.Items.Add("Monday")
ListBox1.Items.Add("Tuesday")
ListBox1.Items.Add("Wednesday")
ListBox1.Items.Add("Thursday")
ListBox1.Items.Add("Friday")
ListBox1.Items.Add("Saturday")
ListBox1.SelectionMode = ListSelectionMode.Multiple
End Sub
Protected Sub Button1_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles Button1.Click
Label1.Text = "you selected : " & ListBox1.SelectedItem.ToString
End Sub
End Class
Conclusion
The ListBox control is commonly used in scenarios where users need to choose items from a predefined list, such as selecting multiple categories, choosing preferences, or picking items from a list of options. Its flexibility and customization options make it a valuable tool for enhancing user interactivity and facilitating data selection in ASP.NET applications.
Related Topics