document.getelementbyid(...) is null
The error "TypeError: document.getelementbyid(...) is null " suggests that the getElementById() function couldn't find an element with the provided ID. This often occurs if the JavaScript code executes before the page is fully loaded, preventing it from locating the element. To resolve this, ensure that you place your JavaScript code after the HTML element's closure or, more generally, before the closing < /body > tag to guarantee that the DOM is ready for manipulation. This approach ensures that the required elements exist when the JavaScript code runs.
Example:When you run the above script , you will the get the error:
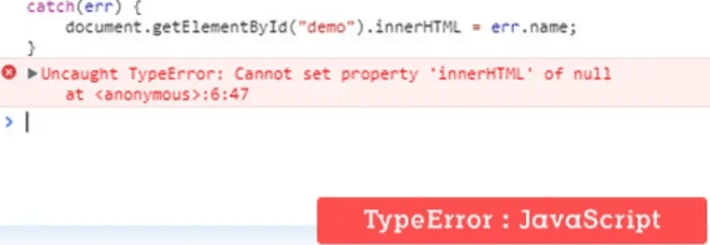
TypeError: document.getElementById(...) is null
If you change the order of the script after the element so that it's defined when getElementById is called.
Example:Placing your script at the bottom of the < /body > element is a common best practice in web development. This ensures that the JavaScript code runs after the HTML content has been loaded and parsed by the browser. This way, when your JavaScript attempts to manipulate or access elements using functions like getElementById(), those elements are already available in the DOM, preventing errors like "null" or "undefined" values. By adhering to this approach, you enhance the reliability and effectiveness of your code.
Alternatively, you can check the null to solve this issue. The standard way to catch null and undefined simultaneously is this:
Null or undefined errors are quite common in JavaScript, and they can lead to unexpected behavior in your code. Utilizing a robust static type checking system like TypeScript, especially with the strict compiler option enabled, can significantly mitigate such errors by catching type-related issues during development. TypeScript's ability to warn about missing or incorrect types can save a lot of debugging time.
In cases where you're not using TypeScript, implementing guard clauses to verify whether objects are undefined before using them is a good practice. This helps prevent your code from encountering runtime errors by ensuring that data is valid before proceeding with any operations. By being proactive in handling potential null or undefined scenarios, you contribute to more stable and error-resistant code.
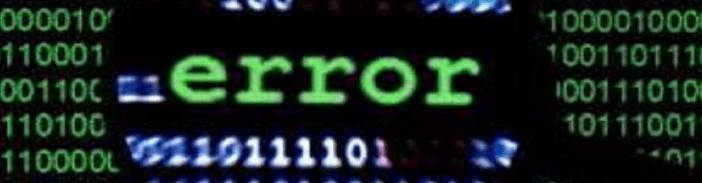
HTML is indeed an interpreted language. The DOMContentLoaded event in JavaScript fires when the initial HTML document has been completely loaded and parsed by the browser. Unlike the load event, it doesn't wait for external resources like stylesheets, images, or frames to finish loading.
When the browser encounters a script in the HTML, it halts parsing to execute the script immediately. This can temporarily block the building of the Document Object Model (DOM). The DOMContentLoaded event typically occurs after these blocking scripts have executed, ensuring that the DOM is ready for manipulation.
The async and defer attributes in external script tags provide ways to optimize this behavior. With async, the script is executed asynchronously without blocking the HTML parsing. With defer, the script is executed after the HTML parsing is complete, just before the DOMContentLoaded event. These attributes help improve page loading performance and user experience by allowing content to be displayed while scripts load in the background.
Conclusion
The error "document.getelementbyid(...) is null" occurs when the getElementById() method is unable to find an element with the provided ID in the Document Object Model (DOM). This commonly happens when JavaScript is executed before the page is fully loaded, making the element inaccessible. Placing the JavaScript code after the relevant HTML element or just before the closing < /body > element helps ensure that the DOM is ready for manipulation, thus preventing this error.
- JavaScript Interview Questions (Part2)
- JavaScript Interview Questions (Part3)
- Is JavaScript a true OOP language?
- Advantages and Disadvantages of JavaScript
- Difference Between JavaScript and ECMAScript?
- What is noscript tag?
- Escaping Special Characters in JavaScript
- What is undefined x 1 in JavaScript?
- Logical operators in JavaScript
- Difference between '=', '==' and '===' operators in JS
- How to loop through objects in JavaScript?
- How to write html code dynamically using JavaScript?
- How to add html elements dynamically with JavaScript?
- How to load another html page from javascript?
- What Is The Disadvantages Using InnerHTML In JavaScript?
- What is Browser Object Model
- How to detect the OS on the client machine in JavaScript?
- Difference between window, document, and screen in Javascript?
- Difference between the substr() and substring() in JavaScript?
- How to replace all occurrences of a string in JavaScript?
- Test a string as a literal and as an object in JavaScript
- What is Associative Array in JavaScript
- What is an anonymous function in JavaScript?
- What is the use of 'bind' method in JavaScript?
- Pure functions Vs. Impure functions in javascript
- Is Javascript a Functional Programming Language?
- What's the Difference Between Class and Prototypal Inheritance?
- Javascript, Pass by Value or Pass by Reference?
- How to prevent modification of an object in Javascript?
- What is 'this' keyword in JavaScript?
- How Does Function Hoisting Work in JavaScript?
- What do mean by NULL in Javascript?
- What does the delete operator do in JavaScript?
- What is the Infinity property used for in Javascript?
- Event bubbling and Event Capturing in JavScript?
- What is "strict mode" and how is it used in JavaScript?
- What is the difference between call and apply in JavaScript
- Entire content of a JavaScript source file in a function block?
- What is an immediately-invoked function expression?
- What is escape & unescape String functions in JavaScript?
- Instanceof operator in JavaScript
- What Are RESTful (REpresentational State Transfer)Web Services?
- What is Unobtrusive JavaScript & Why it's Important?
- What Does JavaScript Void(0) Mean?
- What are JavaScript Cookies?
- Difference between Client side JavaScript and Server side JavaScript
- Uncaught TypeError: Cannot read property of undefined In JavaScript
- Null and Undefined in JavaScript