Null Vs. Undefined in JavaScript
In JavaScript, both null and undefined represent the absence of a value. However, they have distinct use cases and behaviors. Let's investigate into the details with examples:
Undefined in JacaScript
- undefined is a primitive value that indicates a variable has been declared but not assigned any value.
- It's the default value for uninitialized variables and function parameters.
- When accessing an object property or array element that doesn't exist, it results in undefined.
var x;
console.log(x); // Output: undefined
function exampleFunc(y) {
console.log(y); // Output: undefined
}
exampleFunc();
var obj = { key: "value" };
console.log(obj.nonExistent); // Output: undefined
Null in JacaScript
- null is an assignment value that represents the intentional absence of any object value.
- It's often used to indicate that a variable should have no value or to reset a variable.
- Unlike undefined, null is explicitly assigned.
var z = null;
console.log(z); // Output: null
var user = null;
console.log(user); // Output: null
var arr = [1, 2, 3];
arr = null;
console.log(arr); // Output: null
Comparing Undefined and Null
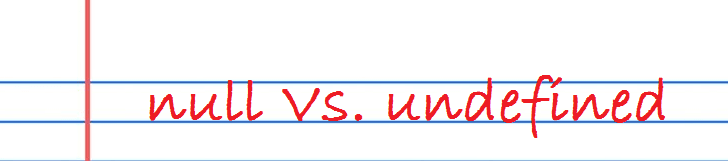
console.log(undefined == null); // Output: true
console.log(undefined === null); // Output: false
Conclusion
JacaScript undefined indicates the lack of an assigned value or the non-existence of a property, while null signifies an intentional absence of value. Understanding the distinction between these two concepts is crucial for writing effective and reliable JavaScript code.
Related Topics
- JavaScript Interview Questions (Part2)
- JavaScript Interview Questions (Part3)
- Is JavaScript a true OOP language?
- Advantages and Disadvantages of JavaScript
- Difference Between JavaScript and ECMAScript?
- What is noscript tag?
- Escaping Special Characters in JavaScript
- What is undefined x 1 in JavaScript?
- Logical operators in JavaScript
- Difference between '=', '==' and '===' operators in JS
- How to loop through objects in JavaScript?
- How to write html code dynamically using JavaScript?
- How to add html elements dynamically with JavaScript?
- How to load another html page from javascript?
- What Is The Disadvantages Using InnerHTML In JavaScript?
- What is Browser Object Model
- How to detect the OS on the client machine in JavaScript?
- Difference between window, document, and screen in Javascript?
- Difference between the substr() and substring() in JavaScript?
- How to replace all occurrences of a string in JavaScript?
- Test a string as a literal and as an object in JavaScript
- What is Associative Array in JavaScript
- What is an anonymous function in JavaScript?
- What is the use of 'bind' method in JavaScript?
- Pure functions Vs. Impure functions in javascript
- Is Javascript a Functional Programming Language?
- What's the Difference Between Class and Prototypal Inheritance?
- Javascript, Pass by Value or Pass by Reference?
- How to prevent modification of an object in Javascript?
- What is 'this' keyword in JavaScript?
- How Does Function Hoisting Work in JavaScript?
- What do mean by NULL in Javascript?
- What does the delete operator do in JavaScript?
- What is the Infinity property used for in Javascript?
- Event bubbling and Event Capturing in JavScript?
- What is "strict mode" and how is it used in JavaScript?
- What is the difference between call and apply in JavaScript
- Entire content of a JavaScript source file in a function block?
- What is an immediately-invoked function expression?
- What is escape & unescape String functions in JavaScript?
- Instanceof operator in JavaScript
- What Are RESTful (REpresentational State Transfer)Web Services?
- What is Unobtrusive JavaScript & Why it's Important?
- What Does JavaScript Void(0) Mean?
- What are JavaScript Cookies?
- Difference between Client side JavaScript and Server side JavaScript
- TypeError: document.getelementbyid(...) is null
- Uncaught TypeError: Cannot read property of undefined In JavaScript