JavaScript Interview Questions (Part2)
What is JavaScript?
JavaScript is a versatile scripting language commonly used for web development. It allows developers to add interactivity and dynamic behavior to websites, making them more engaging and user-friendly.
Which company developed JavaScript?
JavaScript was developed by Netscape Communications Corporation, which is now known as Mozilla Corporation.
What is the difference between JavaScript and JScript?
JavaScript and JScript are both scripting languages, but JavaScript is primarily associated with web browsers, while JScript is Microsoft's implementation of the ECMAScript specification and is used in environments like Internet Explorer.
What is the scope of variables in JavaScript?
JavaScript has both global and function-level scope for variables. Variables declared outside of functions have global scope, while those declared within functions have function-level scope.
Is JavaScript case sensitive? Give an example?
Yes, JavaScript is case sensitive. For example, myVariable and myvariable would be treated as two separate variables.
Can JavaScript code be broken into different lines?
Yes, JavaScript code can be broken into different lines for better readability. Semicolons are used to terminate statements, and line breaks are generally ignored.
Which keyword is used to print text on the screen?
The console.log() function is commonly used to print text and values to the console for debugging purposes in JavaScript.
Which symbol is used for comments in JavaScript?
Double slashes (//) are used for single-line comments, and /* */ is used for multi-line comments in JavaScript.
Can I declare a variable as CONSTANT in JavaScript?
JavaScript doesn't have a built-in constant keyword, but you can achieve constant behavior by declaring a variable with uppercase letters and treating it as constant conventionally.
What is variable typing?
Variable typing refers to whether a programming language enforces a specific data type for a variable or allows the variable's data type to change dynamically during runtime. JavaScript is dynamically typed, meaning variables can change their data type as needed.
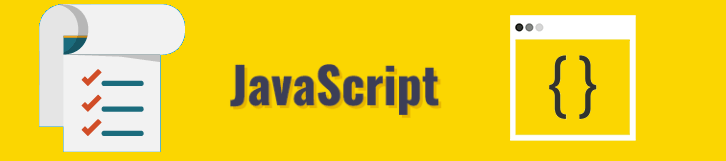
What is the === operator?
The === operator in JavaScript is the strict equality operator. It compares both value and data type, returning true if they are identical and false otherwise.
What is the difference between null and undefined in JavaScript?
null is a value that represents the intentional absence of any object value, while undefined indicates that a variable has been declared but has not been assigned a value.
Between JavaScript and an ASP script, which is faster?
JavaScript is generally faster because it's executed on the client side directly in the browser, while ASP scripts are executed on the server side and generate HTML to be sent to the client.
How to disable an HTML object?
You can disable an HTML object using the disabled attribute. For example, document.getElementById("myButton").disabled = true; would disable a button.
How do you access an element in an HTML document with JavaScript?
You can use methods like getElementById, getElementsByClassName, getElementsByTagName, or newer methods like querySelector and querySelectorAll to access elements in the DOM using JavaScript.
What is the use of the window object?
The window object represents the browser window or frame and provides access to various properties and methods related to the browser environment.
What does the "Access is Denied" IE error mean?
The "Access is Denied" error in Internet Explorer typically occurs when trying to access content from a different domain or subdomain due to the Same Origin Policy restrictions.
What is the screen object in JavaScript?
The screen object in JavaScript provides information about the user's screen, such as its dimensions and color depth.
- JavaScript Interview Questions (Part3)
- Is JavaScript a true OOP language?
- Advantages and Disadvantages of JavaScript
- Difference Between JavaScript and ECMAScript?
- What is noscript tag?
- Escaping Special Characters in JavaScript
- What is undefined x 1 in JavaScript?
- Logical operators in JavaScript
- Difference between '=', '==' and '===' operators in JS
- How to loop through objects in JavaScript?
- How to write html code dynamically using JavaScript?
- How to add html elements dynamically with JavaScript?
- How to load another html page from javascript?
- What Is The Disadvantages Using InnerHTML In JavaScript?
- What is Browser Object Model
- How to detect the OS on the client machine in JavaScript?
- Difference between window, document, and screen in Javascript?
- Difference between the substr() and substring() in JavaScript?
- How to replace all occurrences of a string in JavaScript?
- Test a string as a literal and as an object in JavaScript
- What is Associative Array in JavaScript
- What is an anonymous function in JavaScript?
- What is the use of 'bind' method in JavaScript?
- Pure functions Vs. Impure functions in javascript
- Is Javascript a Functional Programming Language?
- What's the Difference Between Class and Prototypal Inheritance?
- Javascript, Pass by Value or Pass by Reference?
- How to prevent modification of an object in Javascript?
- What is 'this' keyword in JavaScript?
- How Does Function Hoisting Work in JavaScript?
- What do mean by NULL in Javascript?
- What does the delete operator do in JavaScript?
- What is the Infinity property used for in Javascript?
- Event bubbling and Event Capturing in JavScript?
- What is "strict mode" and how is it used in JavaScript?
- What is the difference between call and apply in JavaScript
- Entire content of a JavaScript source file in a function block?
- What is an immediately-invoked function expression?
- What is escape & unescape String functions in JavaScript?
- Instanceof operator in JavaScript
- What Are RESTful (REpresentational State Transfer)Web Services?
- What is Unobtrusive JavaScript & Why it's Important?
- What Does JavaScript Void(0) Mean?
- What are JavaScript Cookies?
- Difference between Client side JavaScript and Server side JavaScript
- TypeError: document.getelementbyid(...) is null
- Uncaught TypeError: Cannot read property of undefined In JavaScript
- Null and Undefined in JavaScript