Uncaught TypeError: Cannot read property of undefined
The error "Uncaught TypeError: Cannot read property of undefined " occurs in JavaScript when you attempt to access a property or method of an object that is undefined. This error indicates that the object you are trying to interact with does not exist or has not been properly defined. Here's a more detailed explanation with examples:
Accessing an Undefined Variable
In this example, person is declared but not assigned a value, so attempting to access the name property of an undefined object results in the error.
Accessing a Property of an Undefined Object
Here, the user object exists, but the age property has not been defined. Trying to access an undefined property leads to the error.
Accessing a Property of a Nested Undefined Object
In this case, the company object exists, but the location object and its city property are not defined, resulting in the error.
How to Handle the Error
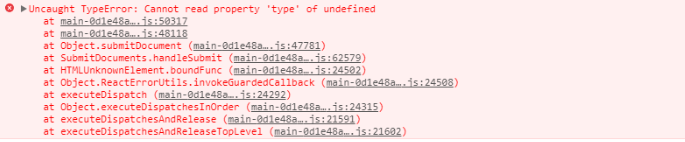
To avoid the "Cannot read property of undefined" error, ensure that the objects you're accessing are properly defined and initialized. You can use conditional checks to handle situations where an object might be undefined.
In this example, we check if person exists and has the age property before trying to access it. This prevents the error and allows you to handle the situation elegantly.
Handling undefined
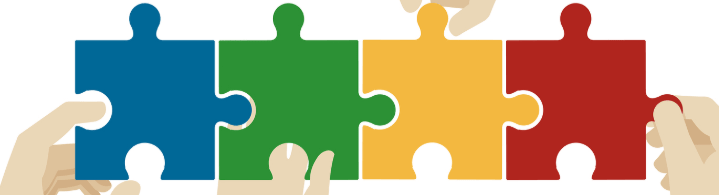
You can handle undefined by using if statement.
JavaScript undefined
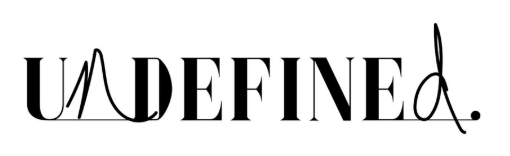
The term "undefined" in JavaScript refers to a variable that has been declared but lacks an assigned value, signifying its uninitialized state. This condition encompasses three distinct facets: the "undefined" type, representing the absence of value assignment; the "undefined" value, which denotes the default state of an undeclared variable; and the option to utilize "undefined" as a variable name, although prudent naming practices would advise against such usage due to its potential to induce confusion.
Also, undefined is of the type undefined:
Moreover, declare a variable then assign undefined to it:
Conclusion
The "Cannot read property of undefined" error occurs when you attempt to interact with properties or methods of objects that are not defined. Properly initializing and checking objects before accessing their properties can help avoid this error.
- JavaScript Interview Questions (Part2)
- JavaScript Interview Questions (Part3)
- Is JavaScript a true OOP language?
- Advantages and Disadvantages of JavaScript
- Difference Between JavaScript and ECMAScript?
- What is noscript tag?
- Escaping Special Characters in JavaScript
- What is undefined x 1 in JavaScript?
- Logical operators in JavaScript
- Difference between '=', '==' and '===' operators in JS
- How to loop through objects in JavaScript?
- How to write html code dynamically using JavaScript?
- How to add html elements dynamically with JavaScript?
- How to load another html page from javascript?
- What Is The Disadvantages Using InnerHTML In JavaScript?
- What is Browser Object Model
- How to detect the OS on the client machine in JavaScript?
- Difference between window, document, and screen in Javascript?
- Difference between the substr() and substring() in JavaScript?
- How to replace all occurrences of a string in JavaScript?
- Test a string as a literal and as an object in JavaScript
- What is Associative Array in JavaScript
- What is an anonymous function in JavaScript?
- What is the use of 'bind' method in JavaScript?
- Pure functions Vs. Impure functions in javascript
- Is Javascript a Functional Programming Language?
- What's the Difference Between Class and Prototypal Inheritance?
- Javascript, Pass by Value or Pass by Reference?
- How to prevent modification of an object in Javascript?
- What is 'this' keyword in JavaScript?
- How Does Function Hoisting Work in JavaScript?
- What do mean by NULL in Javascript?
- What does the delete operator do in JavaScript?
- What is the Infinity property used for in Javascript?
- Event bubbling and Event Capturing in JavScript?
- What is "strict mode" and how is it used in JavaScript?
- What is the difference between call and apply in JavaScript
- Entire content of a JavaScript source file in a function block?
- What is an immediately-invoked function expression?
- What is escape & unescape String functions in JavaScript?
- Instanceof operator in JavaScript
- What Are RESTful (REpresentational State Transfer)Web Services?
- What is Unobtrusive JavaScript & Why it's Important?
- What Does JavaScript Void(0) Mean?
- What are JavaScript Cookies?
- Difference between Client side JavaScript and Server side JavaScript
- TypeError: document.getelementbyid(...) is null
- Null and Undefined in JavaScript