Convert bytes to string in Python
You can convert bytes to string using decode() method:
In Python, the default encoding used for decoding bytes objects to strings is UTF-8. This means that when you call the decode() method on a bytes object with no arguments, it will assume that the byte values are encoded using UTF-8 and will attempt to decode them accordingly.
In Python, you can convert bytes to string using several methods:
- Using the decode() method
- Using the str() method
- Using the bytes() method
- Using the bytearray() method
- Using map() function
- Using pandas
Let's take a look at each method in detail:
Using the decode() method
The most common method for converting bytes to a string in Python is to use the decode() method. The decode() method converts bytes to a string by decoding the byte sequence using a specified encoding.
In the above example, first defined a byte sequence 'b' and then decoded it to a string using the decode() method. Also, specified the encoding as 'utf-8', which is the most commonly used encoding for text in Python. The resulting string is assigned to the variable 'myS'.
Using the str() function
Another way to convert bytes to a string in Python is to use the str() function. The str() function converts any object to a string, including bytes.
In the above example, first defined a byte sequence 'b' and then converted it to a string using the str() function. Then specified the encoding as 'utf-8'. The resulting string is assigned to the variable 'myS'.
Using the bytes() method
You can also convert bytes to a string using the bytes() method. This method creates a bytes object from a string, and then converts it back to a string using the decode() method.
In the above example, first defined a byte sequence 'b' and then created a bytes object using the bytes() method. Then decoded the bytes object to a string using the decode() method, and assigned the resulting string to the variable 'myS'.
Using the bytearray() method
You can also convert bytes to a string using the bytearray() method. This method creates a bytearray object from a bytes object, and then converts it back to a string using the decode() method.
In the above example, first defined a byte sequence 'b' and then created a bytearray object using the bytearray() method. Then decoded the bytearray object to a string using the decode() method, and assigned the resulting string to the variable 's'.
Using map() function

If you have a list of integers representing byte values and you want to convert them to a string without using the b prefix, you can use the map() function along with the chr() function.
In the above example, first defined a list of integers representing byte values. Then used the map() function along with the chr() function to convert each integer to its corresponding ASCII character. The resulting characters are then joined together into a single string using the ''.join() method. Finally, the resulting string is assigned to the variable 's' and printed.
Note that this method assumes that the integers in the list represent ASCII characters. If you're working with non-ASCII characters, you'll need to use an appropriate encoding to convert the bytes to a string.
Using pandas
You can also convert bytes to a string in Python using the pandas library. The pandas library provides a function called "to_string()" that can be used to convert bytes to a string.
In the above example, first imported the pandas library. Then defined a bytes object 'b'. Next, we created a pandas DataFrame with a single column containing the bytes object. Then used the apply() method to apply a lambda function to each element of the 'Bytes' column, which converts the bytes object to a string using the decode() method with the 'utf-8' encoding. Finally, used the to_string() method to convert the resulting Series to a string, and assigned the resulting string to the variable 's' and printed it.
Note that the pandas library is primarily used for data analysis and manipulation, so this method may be useful if you already have a pandas DataFrame containing bytes objects that you want to convert to strings. If you're just working with a single bytes object, using one of the other methods mentioned in the previous answer would be more straightforward.
These are the six methods for converting bytes to a string in Python. You can choose any method that you find most convenient for your use case.
What happened when you Convert bytes to string in Python
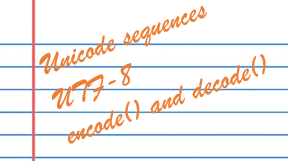
When you convert a bytes object to a string in Python, you are essentially decoding the byte values in the bytes object to their corresponding character representation. This process is called decoding or "stringifying" the bytes object.
In Python, the default encoding used for decoding bytes objects to strings is UTF-8. This means that when you call the decode() method on a bytes object with no arguments, it will assume that the byte values are encoded using UTF-8 and will attempt to decode them accordingly.
If the byte values in the bytes object are not valid UTF-8 encoded characters, the decode() method will raise a UnicodeDecodeError. In this case, you will need to specify a different encoding that matches the encoding used to encode the byte values.
It's worth noting that not all bytes objects can be successfully decoded to strings. For example, if a bytes object contains binary data, attempting to decode it to a string will likely result in unexpected and possibly undesirable behavior.
Conclusion
When you convert bytes to a string, you are converting a sequence of bytes to a sequence of characters, which can be more human-readable and easier to work with in many cases.
- Python Interview Questions (Part 2)
- Python Interview Questions (Part 3)
- What is python used for?
- Is Python interpreted, or compiled, or both?
- Explain how python is interpreted
- How do I install pip on Windows?
- How do you protect Python source code?
- What are the disadvantages of the Python?
- How would you achieve web scraping in Python?
- How to Python Script executable on Unix
- What is the difference between .py and .pyc files?
- What is __init__.py used for in Python?
- What does __name__=='__main__' in Python mean?
- What is docstring in Python?
- What is the difference between runtime and compile time?
- How to use *args and **kwargs in Python
- Purpose of "/" and "//" operator in python?
- What is the purpose pass statement in python?
- Why isn't there a switch or case statement in Python?
- How does the ternary operator work in Python?
- What is the purpose of "self" in Python
- How do you debug a program in Python?
- What are literals in python?
- Is Python call-by-value or call-by-reference?
- What is the process of compilation and Loading in python?
- Global and Local Variables in Python
- Static analysis tools in Python
- What does the 'yield' keyword do in Python?
- Python Not Equal Operator (!=)
- What is the difference between 'is' and '==' in python
- What is the difference between = and == in Python?
- How are the functions help() and dir() different?
- What is the python keyword "with" used for?
- Why isn't all memory freed when CPython exits
- Difference between Mutable and Immutable in Python
- Python Split Regex: How to use re.split() function?
- Accessor and Mutator methods in Python
- How to Implement an 'enum' in Python
- What is Object in Python?
- How to determine the type of instance and inheritance in Python
- Python Inheritance
- How is Inheritance and Overriding methods are related?
- How can you create a copy of an object in Python?
- Class Attributes vs Instance Attributes in Python
- Static class variables in Python
- Difference between @staticmethod and @classmethod in Python
- How to Get a List of Class Attributes in Python
- Does Python supports interfaces like in Java or C#?
- How To Work with Unicode strings in Python
- Difference between lists and tuples in Python?
- What are differences between List and Dictionary in Python
- Different file processing modes supported by Python
- Python append to a file
- Difference Between Multithreading vs Multiprocessing in Python
- Is there any way to kill a Thread in Python?
- What is the use of lambda in Python?
- What is map, filter and reduce in python?
- Is monkey patching considered good programming practice?
- What is "typeerror: 'module' object is not callable"
- Python: TypeError: unhashable type: 'list'
- What are metaclasses in Python?