Not Equal operator in Python
In Python, the "not equal" operator is used to compare two values and determine whether they are not equal to each other. It is represented by the symbol !=. The result of the comparison is a Boolean value: True if the values are not equal, and False if the values are equal.
Numeric Comparison
In this example, x is not equal to y, so the result is True.
String Comparison
Here, the strings "Alice" and "Bob" are not equal, so the result is True.
List Comparison
In this case, the lists list1 and list2 are not equal because they have different elements at the third position.
Boolean Comparison
Here, bool1 is not equal to bool2, so the result is True.
Mixed Data Types
Even though the values number and text appear similar, they are of different types (integer and string), so the result is True.
Comparison with None
In this example, value is None, which is not equal to the integer 10.
Python Comparison Operators
A comparison operator , also called python relational operator, compares the values on both sides of the operator to classify the relation between them as either true or false .
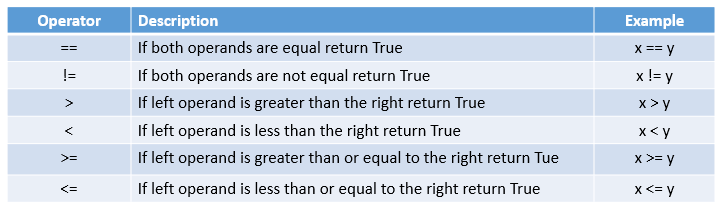
Conclusion
Remember that the "not equal" operator only checks for inequality between two values. If you want to check for both inequality and the data types being the same, you would need to use more complex comparisons, like using the != operator and additionally comparing the types using the type() function.
- Python Interview Questions (Part 2)
- Python Interview Questions (Part 3)
- What is python used for?
- Is Python interpreted, or compiled, or both?
- Explain how python is interpreted
- How do I install pip on Windows?
- How do you protect Python source code?
- What are the disadvantages of the Python?
- How would you achieve web scraping in Python?
- How to Python Script executable on Unix
- What is the difference between .py and .pyc files?
- What is __init__.py used for in Python?
- What does __name__=='__main__' in Python mean?
- What is docstring in Python?
- What is the difference between runtime and compile time?
- How to use *args and **kwargs in Python
- Purpose of "/" and "//" operator in python?
- What is the purpose pass statement in python?
- Why isn't there a switch or case statement in Python?
- How does the ternary operator work in Python?
- What is the purpose of "self" in Python
- How do you debug a program in Python?
- What are literals in python?
- Is Python call-by-value or call-by-reference?
- What is the process of compilation and Loading in python?
- Global and Local Variables in Python
- Static analysis tools in Python
- What does the 'yield' keyword do in Python?
- What is the difference between 'is' and '==' in python
- What is the difference between = and == in Python?
- How are the functions help() and dir() different?
- What is the python keyword "with" used for?
- Why isn't all memory freed when CPython exits
- Difference between Mutable and Immutable in Python
- Python Split Regex: How to use re.split() function?
- Accessor and Mutator methods in Python
- How to Implement an 'enum' in Python
- What is Object in Python?
- How to determine the type of instance and inheritance in Python
- Python Inheritance
- How is Inheritance and Overriding methods are related?
- How can you create a copy of an object in Python?
- Class Attributes vs Instance Attributes in Python
- Static class variables in Python
- Difference between @staticmethod and @classmethod in Python
- How to Get a List of Class Attributes in Python
- Does Python supports interfaces like in Java or C#?
- How To Work with Unicode strings in Python
- Difference between lists and tuples in Python?
- What are differences between List and Dictionary in Python
- Different file processing modes supported by Python
- Python append to a file
- Difference Between Multithreading vs Multiprocessing in Python
- Is there any way to kill a Thread in Python?
- What is the use of lambda in Python?
- What is map, filter and reduce in python?
- Is monkey patching considered good programming practice?
- What is "typeerror: 'module' object is not callable"
- Python: TypeError: unhashable type: 'list'
- How to convert bytes to string in Python?
- What are metaclasses in Python?