Python Metaclasses
Python is an object-oriented language that simplifies working with classes. A class in Python defines specific behaviors for its instances, which are objects in Python. These class instances are created using the class as a blueprint. Similarly, a metaclass in Python describes behaviors for its instances, which are classes. A metaclass serves as the blueprint for the class itself, similar to how a class serves as the blueprint for instances of that class. The default metaclass is "type," and all metaclasses must inherit from the "type" metaclass.
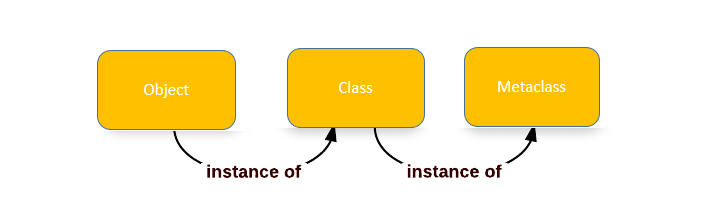
Class-Factory
A metaclass serves primarily as a class factory. When you instantiate an object by invoking the class, Python generates a new class by utilizing the metaclass. By complementing the standard init and new methods, metaclasses offer the capability to incorporate additional functionalities during class creation, such as registering the freshly created class within a registry or substituting the class with an entirely different entity.
There are numerous use cases for metaclasses . Here are few:
- Automatic property creation
- Automatically adding new methods
- Registering classes at creation time
- Logging and profiling
- Interface checking
Metaclass's __new__() and __init__()
- __new__(): It's a method which is called before __init__(). It creates the object and return it. We can overide this method to control how the objects are created.
- __init__(): This method just initialize the created object passed as parameter.
Metaclasses can be defined in one of the two ways shown below.
To exercise control over the construction and setup of classes within the metaclass, you can implement the metaclass's new method and/or init constructor. In practice, many metaclasses tend to override only one of these methods. Similar to a regular class, a metaclass possesses a new method responsible for generating the class instance and an init method that personalizes the instance. Additionally, if you intend to return an entity other than a freshly established class of the specific type, you must utilize new.
When to use Metaclasses?
In practical scenarios, the necessity for utilizing metaclasses is relatively infrequent. Typically, metaclasses come into play when there's a requirement for dynamic behavior that transcends the object level and is instead defined at the class level.
A Simple metaclass
We can use type directly to make a class, without any class statement. It can be called in following ways:
- When called with only one argument, it returns the type.
- When called with three parameters, it creates a class. Following arguments are passed to it:
- Class name
- Tuple having base classes inherited by class
- Class Dictionary
It's because the function type is in fact a metaclass. type is the metaclass Python uses to create all classes behind the scenes.
The class statement isn't just syntactic sugar, it does some extra things, like setting an adequate __qualname__ and __doc__ properties or calling __prepare__ .
The __prepare__ method
The prepare method is invoked prior to executing the class body, and its role is to yield a dictionary-like object which serves as the local namespace for the entirety of the code within the class body. When the metaclass has a prepare attribute, it's invoked with the arguments namespace = metaclass.prepare(name, bases, **kwds). Conversely, in cases where the metaclass lacks a prepare attribute, the class namespace gets initialized as an empty ordered mapping. This feature was introduced in Python 3.0.

Creating custom Metaclass
The primary objective of a metaclass is to automatically modify a class during its creation. This practice is typically applied to APIs, where the goal is to generate classes that align with the present context. To craft a custom metaclass, it should inherit from the type metaclass and commonly override:
custom metaclass:And then we can use it
Conclusion
Python metaclasses are a powerful feature that allows you to control the creation and behavior of classes. They provide a way to customize class creation and can be used to implement dynamic behaviors that operate at the class level, offering advanced capabilities beyond traditional class inheritance.
- Python Interview Questions (Part 2)
- Python Interview Questions (Part 3)
- What is python used for?
- Is Python interpreted, or compiled, or both?
- Explain how python is interpreted
- How do I install pip on Windows?
- How do you protect Python source code?
- What are the disadvantages of the Python?
- How would you achieve web scraping in Python?
- How to Python Script executable on Unix
- What is the difference between .py and .pyc files?
- What is __init__.py used for in Python?
- What does __name__=='__main__' in Python mean?
- What is docstring in Python?
- What is the difference between runtime and compile time?
- How to use *args and **kwargs in Python
- Purpose of "/" and "//" operator in python?
- What is the purpose pass statement in python?
- Why isn't there a switch or case statement in Python?
- How does the ternary operator work in Python?
- What is the purpose of "self" in Python
- How do you debug a program in Python?
- What are literals in python?
- Is Python call-by-value or call-by-reference?
- What is the process of compilation and Loading in python?
- Global and Local Variables in Python
- Static analysis tools in Python
- What does the 'yield' keyword do in Python?
- Python Not Equal Operator (!=)
- What is the difference between 'is' and '==' in python
- What is the difference between = and == in Python?
- How are the functions help() and dir() different?
- What is the python keyword "with" used for?
- Why isn't all memory freed when CPython exits
- Difference between Mutable and Immutable in Python
- Python Split Regex: How to use re.split() function?
- Accessor and Mutator methods in Python
- How to Implement an 'enum' in Python
- What is Object in Python?
- How to determine the type of instance and inheritance in Python
- Python Inheritance
- How is Inheritance and Overriding methods are related?
- How can you create a copy of an object in Python?
- Class Attributes vs Instance Attributes in Python
- Static class variables in Python
- Difference between @staticmethod and @classmethod in Python
- How to Get a List of Class Attributes in Python
- Does Python supports interfaces like in Java or C#?
- How To Work with Unicode strings in Python
- Difference between lists and tuples in Python?
- What are differences between List and Dictionary in Python
- Different file processing modes supported by Python
- Python append to a file
- Difference Between Multithreading vs Multiprocessing in Python
- Is there any way to kill a Thread in Python?
- What is the use of lambda in Python?
- What is map, filter and reduce in python?
- Is monkey patching considered good programming practice?
- What is "typeerror: 'module' object is not callable"
- Python: TypeError: unhashable type: 'list'
- How to convert bytes to string in Python?