typeerror: 'module' object is not callable
The error message "TypeError: 'module' object is not callable " is triggered when confusion arises between a module name and a class name. The issue typically originates from the import statement, where a module is imported instead of a class. This confusion can occur due to identical names between the module and the class, leading to the error.
If you have a class MyClass in a file called MyClass.py , then you should write:
How to fix typeerror: 'module' object is not callable
The following Python example shows, a file named MyClass.py contains a class named MyClass. When you import the module "MyClass" into another Python file named sample.py, Python recognizes the imported module as "MyClass," but it doesn't directly perceive the class named "MyClass" declared within that module. This situation can result in confusion when attempting to access the class's attributes or methods in the importing file.
When you run the sample.py , you will get the following error.

In Python, a script is essentially a module whose name corresponds to the filename. Thus, initiating your file MyClass.py with the line import MyClass inadvertently creates a circular reference in the module structure. This circularity can lead to complications and should be avoided to ensure proper module functioning.
'module' object is not callable
You can fix this error by change the import statement in the sample.py sample.py
Here you can see, when you changed the import statement to from MyClass import MyClass , you will get the error fixed.
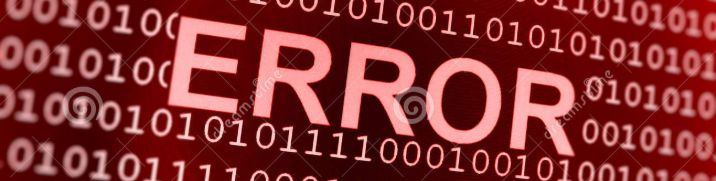
In Python, all entities such as functions, methods, modules, and classes are objects, and methods are akin to other attributes. Consequently, there exists no distinct namespaces exclusively for methods. Therefore, when an instance attribute is established, it supersedes a class attribute sharing the same name. To circumvent this, a straightforward remedy is to assign distinct names to attributes.
Conclusion
The "TypeError: 'module' object is not callable " error arises when you mistakenly attempt to call a module as if it were a function or callable object. This often occurs due to confusion between a module's name and the name of a class or function within it, leading to improper usage.
- Python Interview Questions (Part 2)
- Python Interview Questions (Part 3)
- What is python used for?
- Is Python interpreted, or compiled, or both?
- Explain how python is interpreted
- How do I install pip on Windows?
- How do you protect Python source code?
- What are the disadvantages of the Python?
- How would you achieve web scraping in Python?
- How to Python Script executable on Unix
- What is the difference between .py and .pyc files?
- What is __init__.py used for in Python?
- What does __name__=='__main__' in Python mean?
- What is docstring in Python?
- What is the difference between runtime and compile time?
- How to use *args and **kwargs in Python
- Purpose of "/" and "//" operator in python?
- What is the purpose pass statement in python?
- Why isn't there a switch or case statement in Python?
- How does the ternary operator work in Python?
- What is the purpose of "self" in Python
- How do you debug a program in Python?
- What are literals in python?
- Is Python call-by-value or call-by-reference?
- What is the process of compilation and Loading in python?
- Global and Local Variables in Python
- Static analysis tools in Python
- What does the 'yield' keyword do in Python?
- Python Not Equal Operator (!=)
- What is the difference between 'is' and '==' in python
- What is the difference between = and == in Python?
- How are the functions help() and dir() different?
- What is the python keyword "with" used for?
- Why isn't all memory freed when CPython exits
- Difference between Mutable and Immutable in Python
- Python Split Regex: How to use re.split() function?
- Accessor and Mutator methods in Python
- How to Implement an 'enum' in Python
- What is Object in Python?
- How to determine the type of instance and inheritance in Python
- Python Inheritance
- How is Inheritance and Overriding methods are related?
- How can you create a copy of an object in Python?
- Class Attributes vs Instance Attributes in Python
- Static class variables in Python
- Difference between @staticmethod and @classmethod in Python
- How to Get a List of Class Attributes in Python
- Does Python supports interfaces like in Java or C#?
- How To Work with Unicode strings in Python
- Difference between lists and tuples in Python?
- What are differences between List and Dictionary in Python
- Different file processing modes supported by Python
- Python append to a file
- Difference Between Multithreading vs Multiprocessing in Python
- Is there any way to kill a Thread in Python?
- What is the use of lambda in Python?
- What is map, filter and reduce in python?
- Is monkey patching considered good programming practice?
- Python: TypeError: unhashable type: 'list'
- How to convert bytes to string in Python?
- What are metaclasses in Python?