TypeError: unhashable type: 'list'
The error message "TypeError: unhashable type: 'list' " typically indicates an attempt to employ a list as a hashable argument. Hashing an unhashable object leads to an error. For example, using a list as a dictionary key is infeasible since lists aren't hashable. The conventional resolution involves converting the list to a tuple.
example
This error shows that the my_dict key [1,2,3] is List and List is not a hashable type in Python . Dictionary keys must be immutable types and list is a mutable type.
Fix: Cast list to a tuple
You'll have to change your list into tuples if you want to put them as keys in your dictionary .
The hash() function is a built-in Python method utilized to generate a distinct numerical value. It can be employed with user-defined objects that remain unaltered after initialization. This characteristic finds significance primarily in the context of dictionary keys.
Examples of hashable objects:
Examples of Unhashable objects:
Tuple and List
While tuples might appear similar to lists, they frequently serve distinct roles and have different applications. Tuples are immutable and typically comprise a heterogeneous sequence of elements accessible through unpacking or indexing. In contrast, lists are mutable and generally consist of homogeneous elements accessed by iteration.
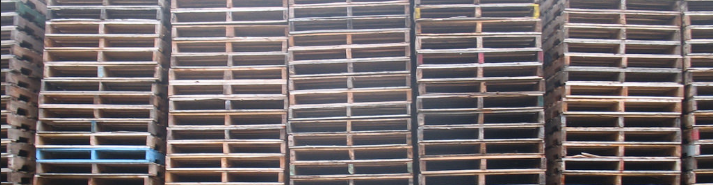
Hashing
Hashing is a fundamental concept in computer science that facilitates the creation of efficient, pseudo-random access data structures for rapid storage and retrieval of substantial data. Immutable objects, those that remain unmodifiable, are deemed hashable, possessing an unchanging unique value. A hashing function takes an object, such as the string "Java," and produces a consistent, fixed-size code—typically an integer.
Conclusion
The "TypeError: unhashable type: 'list'" error occurs when attempting to use a list as a hashable object. Lists are mutable and lack the properties necessary for reliable hashing, making them unsuitable as dictionary keys or elements in sets. To resolve this, consider using tuples, which are immutable and can serve as hashable alternatives in such contexts.
- Python Interview Questions (Part 2)
- Python Interview Questions (Part 3)
- What is python used for?
- Is Python interpreted, or compiled, or both?
- Explain how python is interpreted
- How do I install pip on Windows?
- How do you protect Python source code?
- What are the disadvantages of the Python?
- How would you achieve web scraping in Python?
- How to Python Script executable on Unix
- What is the difference between .py and .pyc files?
- What is __init__.py used for in Python?
- What does __name__=='__main__' in Python mean?
- What is docstring in Python?
- What is the difference between runtime and compile time?
- How to use *args and **kwargs in Python
- Purpose of "/" and "//" operator in python?
- What is the purpose pass statement in python?
- Why isn't there a switch or case statement in Python?
- How does the ternary operator work in Python?
- What is the purpose of "self" in Python
- How do you debug a program in Python?
- What are literals in python?
- Is Python call-by-value or call-by-reference?
- What is the process of compilation and Loading in python?
- Global and Local Variables in Python
- Static analysis tools in Python
- What does the 'yield' keyword do in Python?
- Python Not Equal Operator (!=)
- What is the difference between 'is' and '==' in python
- What is the difference between = and == in Python?
- How are the functions help() and dir() different?
- What is the python keyword "with" used for?
- Why isn't all memory freed when CPython exits
- Difference between Mutable and Immutable in Python
- Python Split Regex: How to use re.split() function?
- Accessor and Mutator methods in Python
- How to Implement an 'enum' in Python
- What is Object in Python?
- How to determine the type of instance and inheritance in Python
- Python Inheritance
- How is Inheritance and Overriding methods are related?
- How can you create a copy of an object in Python?
- Class Attributes vs Instance Attributes in Python
- Static class variables in Python
- Difference between @staticmethod and @classmethod in Python
- How to Get a List of Class Attributes in Python
- Does Python supports interfaces like in Java or C#?
- How To Work with Unicode strings in Python
- Difference between lists and tuples in Python?
- What are differences between List and Dictionary in Python
- Different file processing modes supported by Python
- Python append to a file
- Difference Between Multithreading vs Multiprocessing in Python
- Is there any way to kill a Thread in Python?
- What is the use of lambda in Python?
- What is map, filter and reduce in python?
- Is monkey patching considered good programming practice?
- What is "typeerror: 'module' object is not callable"
- How to convert bytes to string in Python?
- What are metaclasses in Python?