ASP.NET GridView Editing
The GridView control provides many built-in capabilities that allow the user to sort, update, delete, select, and page through items in the control.
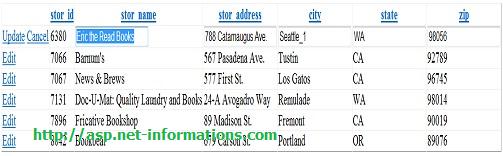
The GridView control facilitates convenient row-by-row editing functionality, enabling users to modify data directly within the control. An editable GridView incorporates an additional column in each row, housing an Edit button. When a user clicks on the Edit button, the corresponding row transitions into an editable state. As a result, the Edit button transforms into Update and Cancel buttons, while the remaining columns become TextBoxes, allowing for easy data manipulation.
Enable editing capabilities
To enable editing capabilities, you can set the AutoGenerateEditButton property of the GridView control to true. This setting triggers the automatic generation of the Edit button within each row, streamlining the editing process for users. Additionally, it is crucial to specify the DataKeyNames property with the primary key of the underlying table. By doing so, the GridView control can track and identify individual rows accurately, ensuring the proper updating of data when the user clicks the Update button.
Enabling editing functionality within the GridView control empowers users to modify column values seamlessly, enhancing the interactivity and flexibility of the displayed data. Users can effortlessly update one or more fields within a row and then save their changes by clicking the Update button, providing a convenient means of data manipulation.
Download Database
In this article I have used Microsoft's Pubs database for sample data. You can download it free from the following link.
DownloadBefore you start to create Edit functionality on GridView in your asp file, you should create a ConnectionString in your web.Config File. Double click the web.config file on the right hand side of the Visual Studio and add the following connectionstring code in that file.
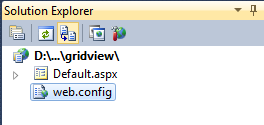
Web.Config File
The following program shows how to update gridview values from an ASP.NET application
Default.aspx
- ASP.NET Simple GridView
- Sorting , Paging and AutoGenerateColumns
- ASP.NET GridView Delete
- DropDownList in GridView
- Create Gridview at runtime
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Asp.Net GridView summary on Footer
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- Create Gridview without database
- GridView from Stored Procedure
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Auto Generate Row Number in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control