Auto Generate Row Number in GridView
The GridView control, as the successor to the DataGrid, offers several enhancements and extended capabilities. While developing GridView controls in ASP.NET, programmers frequently encounter the need to display row numbers within the GridView.
Gridview row index number
Displaying row numbers in a GridView can provide valuable information and improve the user experience. It allows users to easily track and reference specific rows within the GridView, facilitating navigation and data comprehension.
To accomplish this, programmers can utilize various techniques. One common approach is to use the RowDataBound event of the GridView control. Within this event handler, the programmer can access each row of the GridView and assign a unique row number value based on the current row's index or position within the GridView.
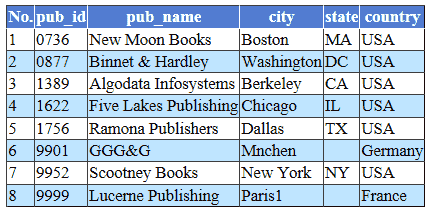
Displaying row numbers in a GridView enhances the usability and clarity of the displayed data. It provides users with a convenient reference point, enabling them to identify and interact with specific rows more effectively. Programmers can implement this feature easily within the ASP.NET GridView control, enhancing the overall functionality and user experience.
Database
In this article I have used Microsoft's Pubs database for sample data. You can download it free from the following link.
DownloadAuto Generate Row Number in GridView
It is a common requirement that GridViews to have the first column just be a number identifying the row. Because of doing this implementation, the user can see the number of rows returned by scrolling to the end of the GridView. By adding a single piece of code you can add a row number column to your asp.net GridView Control.
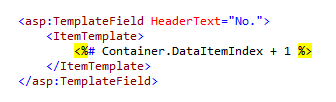
The following source code show how to create a row number column field to your Asp.Net GridView
Default.aspx- ASP.NET Simple GridView
- Sorting , Paging and AutoGenerateColumns
- ASP.NET GridView Editing
- ASP.NET GridView Delete
- DropDownList in GridView
- Create Gridview at runtime
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Asp.Net GridView summary on Footer
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- Create Gridview without database
- GridView from Stored Procedure
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control