Asp.Net GridView summary on Footer
In the scope of Asp.Net GridView, the need to present summary data arises frequently, particularly when generating reports. A key requirement in this regard is to display the total value derived from all rows within a dedicated Footer row. Fortunately, incorporating this vital footer row into the GridView is a seamless process.
Footer template
Developers can tap into the GridView control's inherent capabilities to effortlessly organize and manipulate tabular data. utilizing functionalities such as the footer template, they can precisely reflect aggregated values derived from the grid rows. This integration of the footer row not only enhances the visual appeal of the GridView but also provides users with a concise summary of the data.
By incorporating a footer row, the GridView imparts a streamlined and structured appearance, enabling users to navigate through the data more efficiently. This feature empowers users to gain a comprehensive understanding of the information presented, facilitating better analysis and decision-making.
Through the use of the footer template and other related functionalities, developers can elevate the usability and visual presentation of the GridView, ensuring a more engaging and informative experience for users.
Database
In this article, we have utilized Microsoft's Pubs database as a reliable source of sample data. To access this invaluable resource, you can easily download it free of charge by following the provided link.
DownloadCalculate Total and display in Gridview
Within the upcoming program, the primary objective entails retrieving data from the STOR table within the esteemed PUBS database and subsequently showcasing the Total Quantity within the footer row. To successfully accomplish this, the inclusion of a FooterTemplate within the GridView becomes imperative as it enables the generation of summary reports in an efficient and organized manner.
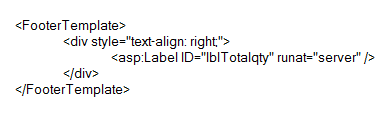
Add gridview totals in the footer
In the code behind, during the RowDataBound event, a crucial operation takes place wherein the quantity is fetched from each individual row, subsequently leading to the calculation of the total quantity.
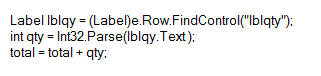
Once the total quantity has been accurately calculated, the program proceeds to assign this value to the lblTotalqty control located within the footer of the GridView.
GridView summary on Footer
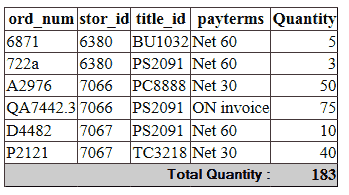
- ASP.NET Simple GridView
- Sorting , Paging and AutoGenerateColumns
- ASP.NET GridView Editing
- ASP.NET GridView Delete
- DropDownList in GridView
- Create Gridview at runtime
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- Create Gridview without database
- GridView from Stored Procedure
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Auto Generate Row Number in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control