Create Gridview at runtime
In Asp.Net one of the most frequently used Web Control is the GridView control. In the previous examples we access data from database using SqlDataSource. So no need of Code behind use to retrieve the data. Also we fixes database , sql and everything before we run the program.
Gridview from SqlDataSource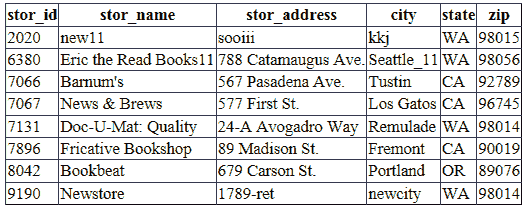
Drag-and-drop functionality
In this article, we will investigate into the implementation of the GridView control for data display purposes. To showcase data in real-time, the initial step involves seamlessly incorporating the GridView onto your ASP.NET page. This can be easily achieved by utilizing the drag-and-drop functionality provided by the ASP.NET development environment. By selecting and placing the DataGrid control onto the desired location within your page, you can establish the foundation for presenting data in a structured and visually appealing manner.
However, it is crucial to note that the configuration and customization of the GridView extend beyond the visual design aspect. To ensure the desired functionality and appearance, various settings and properties of the GridView control need to be appropriately defined in the code-behind file. This entails programming the necessary logic and data source connections to populate the GridView dynamically. After drag and drop the gridview, your design page look like the following image :
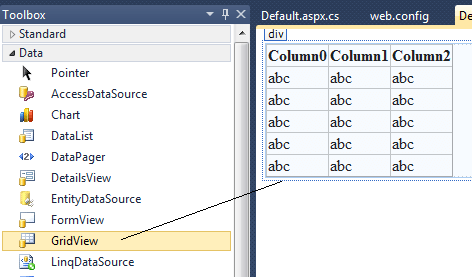
After drag and drop the gridview on the form, we go to the code behind section and write the code for accessing data from database and display on Gridview.
Database
In this article we are using Microsoft's Pubs database for retrieving data. You can download Pubs database from the following link:
DownloadIn this program we are displaying the data from Stores table in the Pubs database. So first create a connection string and then create the sql statement for retrieving the data.
After retrieving the data in Dataset, you should set the data source for gridview as Dataset.
From the following source code, you can create a gridview and fill the data at runtime.
Default.aspx
C# Source Code
VB.Net Source Code
- ASP.NET Simple GridView
- Sorting , Paging and AutoGenerateColumns
- ASP.NET GridView Editing
- ASP.NET GridView Delete
- DropDownList in GridView
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Asp.Net GridView summary on Footer
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- Create Gridview without database
- GridView from Stored Procedure
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Auto Generate Row Number in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control