Sorting , Paging and AutoGenerateColumns
The GridView control offers a comprehensive array of built-in functionalities, empowering users with the ability to efficiently perform sorting, updating, deleting, selecting, and paging operations on the items contained within the control. These capabilities provide a seamless and intuitive user experience, allowing for easy manipulation and management of data displayed within the GridView. Whether it's organizing data based on specific criteria, modifying existing records, removing unwanted entries, selecting particular items of interest, or navigating through multiple pages of content, the GridView control streamlines these tasks, enhancing user productivity and interaction.
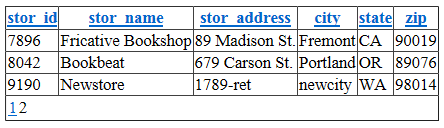
Download Database
In this article I have used Microsoft's Pubs database for sample data. You can download it free from the following link.
DownloadBefore you start to generate GridView in your asp file, you should create a ConnectionString in your web.Config File. Double click the web.config file on the right hand side of the Visual Studio and add the following connectionstring code in that file.
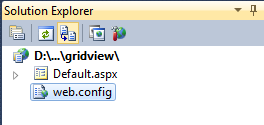
Web.Config File
Within the GridView control, each column is represented by a DataControlField object. By default, the AutoGenerateColumns property is set to true, which allows the GridView control to automatically generate columns based on the data source. This automatic generation is convenient when you want to quickly display all available fields.
However, if you prefer more control over the appearance and arrangement of columns, you can manually specify the column fields by setting the AutoGenerateColumns property to false. This gives you the flexibility to define the columns explicitly and customize their properties according to your requirements. By disabling the automatic generation of columns, you can tailor the GridView layout to match your desired presentation style and achieve a more personalized and precise representation of your data.
Sorting allows the user to sort the items in the GridView control with respect to a specific column by clicking on the column's header. To enable sorting, set the AllowSorting property to true.
To enhance the usability and manageability of large datasets, the GridView control offers a convenient paging feature. Instead of displaying all records from the data source simultaneously, the GridView control can automatically divide them into separate pages.
To enable paging functionality, you can set the AllowPaging property of the GridView control to true. By doing so, the GridView control will automatically generate pagination controls, such as next and previous buttons or page numbers, allowing users to navigate through different pages of data.
This feature is particularly useful when dealing with a significant amount of information, as it improves performance by reducing the load on the page and enhances user experience by presenting data in a more organized and digestible manner. With paging enabled, users can easily browse through different pages of records within the GridView control, simplifying navigation and ensuring efficient data retrieval.
Also we can set how many rows we want to see in a page.
The following ASP.NET program shows how to enable sorting and paging in a GridView with a custom defined column fields.
Default.aspx
- Create Gridview at runtime
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Asp.Net GridView summary on Footer
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- Create Gridview without database
- GridView from Stored Procedure
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Auto Generate Row Number in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control