GridView from Stored Procedure
In this article, you can see how to populate a gridview from stored procedure.
Stored Procedure
Stored procedures provide a secure and efficient way to encapsulate Transact-SQL statements, offering improved performance, code reusability, and enhanced security. They are a valuable tool for database developers and administrators to enhance the functionality and manageability of database systems.
Database
In this article I have used Microsoft's Pubs database for sample data. You can download it free from the following link.
DownloadHow to create a Stored Procedure ?
The following SQL statements will create a Store Procedure.
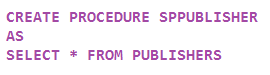
The above code create a store procedure named as 'SPPUBLISHER' and it execute SQL statement that select data of all publishers from publishers table from the PUB database.
Grid View Binding using Stored Procedure
You can retrieve data from database using this Store Procedure and display it in a GridView.
To call a stored procedure from C# application, set the CommandType of the Command object to StoredProcedure.
Next step is to inform the your Store Procedure name to the Command Object.
The rest of the things are same as SQl Query string.

Finally you can set GridView DataSource as your Dataset.

- ASP.NET Simple GridView
- Sorting , Paging and AutoGenerateColumns
- ASP.NET GridView Editing
- ASP.NET GridView Delete
- DropDownList in GridView
- Create Gridview at runtime
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Asp.Net GridView summary on Footer
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- Create Gridview without database
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Auto Generate Row Number in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control