Gridview without database
The following program show how to create a GridView without taking values from database.
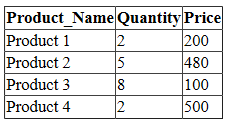
Here the program create a DataTable and create the data structure.

After creating the data structure, it adds rows in the DataTable.
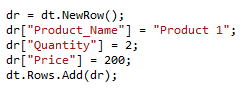
Finally the DataTable add in the Dataset and make this dataset as GridView's DataSource.
ds.Tables.Add(dt);
GridView1.DataSource = ds.Tables[0];
GridView1.DataBind();
Default.aspx
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</div>
</form>
</body>
</html>
C# Source Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
loadDataTable();
}
private void loadDataTable()
{
DataSet ds = new DataSet();
DataTable dt;
DataRow dr;
DataColumn pName;
DataColumn pQty;
DataColumn pPrice;
int i = 0;
dt = new DataTable();
pName = new DataColumn("Product_Name", Type.GetType("System.String"));
pQty = new DataColumn("Quantity", Type.GetType("System.Int32"));
pPrice = new DataColumn("Price", Type.GetType("System.Int32"));
dt.Columns.Add(pName);
dt.Columns.Add(pQty);
dt.Columns.Add(pPrice);
dr = dt.NewRow();
dr["Product_Name"] = "Product 1";
dr["Quantity"] = 2;
dr["Price"] = 200;
dt.Rows.Add(dr);
dr = dt.NewRow();
dr["Product_Name"] = "Product 2";
dr["Quantity"] = 5;
dr["Price"] = 480;
dt.Rows.Add(dr);
dr = dt.NewRow();
dr["Product_Name"] = "Product 3";
dr["Quantity"] = 8;
dr["Price"] = 100;
dt.Rows.Add(dr);
dr = dt.NewRow();
dr["Product_Name"] = "Product 4";
dr["Quantity"] = 2;
dr["Price"] = 500;
dt.Rows.Add(dr);
ds.Tables.Add(dt);
GridView1.DataSource = ds.Tables[0];
GridView1.DataBind();
}
}
VB.Net Source Code
Imports System.Drawing
Imports System.Data.SqlClient
Imports System.Data
Partial Class _Default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
loadDataTable()
End Sub
Private Sub loadDataTable()
Dim ds As New DataSet()
Dim dt As DataTable
Dim dr As DataRow
Dim pName As DataColumn
Dim pQty As DataColumn
Dim pPrice As DataColumn
Dim i As Integer = 0
dt = New DataTable()
pName = New DataColumn("Product_Name", Type.[GetType]("System.String"))
pQty = New DataColumn("Quantity", Type.[GetType]("System.Int32"))
pPrice = New DataColumn("Price", Type.[GetType]("System.Int32"))
dt.Columns.Add(pName)
dt.Columns.Add(pQty)
dt.Columns.Add(pPrice)
dr = dt.NewRow()
dr("Product_Name") = "Product 1"
dr("Quantity") = 2
dr("Price") = 200
dt.Rows.Add(dr)
dr = dt.NewRow()
dr("Product_Name") = "Product 2"
dr("Quantity") = 5
dr("Price") = 480
dt.Rows.Add(dr)
dr = dt.NewRow()
dr("Product_Name") = "Product 3"
dr("Quantity") = 8
dr("Price") = 100
dt.Rows.Add(dr)
dr = dt.NewRow()
dr("Product_Name") = "Product 4"
dr("Quantity") = 2
dr("Price") = 500
dt.Rows.Add(dr)
ds.Tables.Add(dt)
GridView1.DataSource = ds.Tables(0)
GridView1.DataBind()
End Sub
End Class
Related Topics
- ASP.NET Simple GridView
- Sorting , Paging and AutoGenerateColumns
- ASP.NET GridView Editing
- ASP.NET GridView Delete
- DropDownList in GridView
- Create Gridview at runtime
- Asp.Net Gridview - Add, Edit and delete
- Gridview export to Excel
- Gridview export to CSV
- Asp.Net GridView summary on Footer
- Subtotal row in Gridview
- SubTotal and GrandTotal in GridView
- GridView from Stored Procedure
- How to create Gridview Popup Form
- How to create a Blank row in gridview
- Nested GridView in ASP.NET
- Freeze Gridview Header row, Scroll in GridView
- Auto Generate Row Number in GridView
- Image between GridView rows
- How to select a row in gridview
- Checkbox in ASP.NET GridView Control