Difference between Abstract class and Interface in Java
Summary:
- An abstract class can have instance variables, whereas an interface cannot.
- A class may inherit from only one abstract class, but it can implement multiple interfaces.
- An abstract class can have concrete (i.e., non-abstract) methods, while an interface can only have abstract methods.
- An abstract class can provide a default implementation of an interface, but an interface cannot provide a default implementation of an abstract class.
Abstract Class Vs Interface
In this section, you will be provided with a comprehensive comparison between the abstract class and interface, highlighting the differences between the two concepts in great detail.
Type of variables
Abstract classes and interfaces can have different types of variables:
An abstract class can have both instance variables (non-static variables declared within the class, associated with objects of the class) and static variables (variables declared using the static keyword, associated with the class as a whole). An abstract class can also have both final and non-final variables. Final variables are variables that once assigned a value, cannot be changed.
An interface can only have static and final/constant variables. The variables in an interface are implicitly public, static, and final, and therefore must be assigned a value when they are declared.
The main difference between abstract classes and interfaces with regards to variables is that an abstract class can have both instance and static variables, both final and non-final, while an interface can only have static and final/constant variables.
Type of methods
In Java, both abstract classes and interfaces can have methods, but there are some differences between the types of methods they can have:
An abstract class can have both abstract methods (methods without a body, meant to be overridden by subclasses) and concrete methods (methods with a body that provide a default implementation).
An interface can only have abstract methods (methods without a body). The implementation of these methods must be provided by the classes that implement the interface.
The main difference between abstract classes and interfaces with regards to methods is that an abstract class can have both abstract and concrete methods, while an interface can only have abstract methods.
Accessibility of Data Members
The accessibility of data members in Object Oriented Programming is affected by both Abstract Classes and Interfaces.
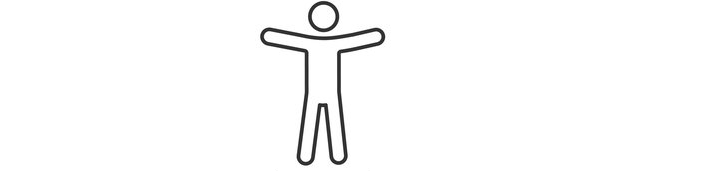
Inheritance, which is typically used in conjunction with Abstract Classes, affects the accessibility of data members by determining the accessibility of the data members in a subclass based on the accessibility of the data members in the parent class. For example, if a parent class has a private data member, it will not be accessible to the subclass. If a parent class has a protected data member, it will be accessible to the subclass. If a parent class has a public data member, it will be accessible to the subclass and to any other class that has access to an instance of the subclass.
Abstraction, achieved through Abstract Classes and Interfaces, does not directly impact the accessibility of data members. The accessibility of data members in a class implementing an interface or extending an abstract class is determined by the accessibility modifiers used in the class definition, independent of the abstraction mechanism.
The accessibility of data members is affected by both Inheritance and Abstraction. Inheritance affects the accessibility of data members by determining the accessibility of the data members in a subclass based on the accessibility of the data members in the parent class. Abstraction does not directly impact the accessibility of data members.
Implementation
An abstract class is a class that contains one or more abstract methods (methods with no implementation). It can provide a partial implementation, with concrete methods that can be shared among subclasses, and abstract methods that must be implemented by the subclasses.
An interface, on the other hand, is a pure abstract class, with only abstract methods (no implementation at all). An interface can be implemented by a class, providing a full implementation for all its abstract methods. A single class can implement multiple interfaces.
In terms of implementation, when a class implements an interface, it must provide a complete implementation for all the abstract methods defined in the interface. When a class extends an abstract class, it must provide implementation for all the abstract methods of the abstract class and any additional abstract methods in its own definition.
Inheritance vs Abstraction
Abstract class and interface both provide the mechanism of abstraction in Object Oriented Programming. An abstract class can contain abstract methods with no implementation and concrete methods with implementation, while an interface contains only abstract methods with no implementation. Both abstract classes and interfaces provide a way to declare common methods that must be implemented by the subclass or implementing class.
Inheritance is the mechanism in OOP where a class inherits the properties and behavior of its parent class. A subclass can extend its parent class and inherit all its fields and methods. Abstract classes can be used as a base class for inheritance, as a subclass can inherit all fields and methods from the abstract class.
Abstraction is the mechanism of hiding the implementation details and exposing only the essential features of an object. This mechanism is achieved through abstract classes and interfaces in OOP.
Multiple Inheritance
Multiple Inheritance is a feature in Object Oriented Programming where a class can inherit from multiple classes.
In class-based languages, multiple inheritance is typically supported through inheritance of classes. However, multiple inheritance can lead to issues with ambiguity and method conflicts, as a subclass may inherit multiple implementations of the same method from different parent classes.
Abstraction does not support multiple inheritance of classes, but it does support multiple inheritance of behavior through the concept of interface implementation. A class can implement multiple interfaces, which can provide multiple implementations for the same behavior. Interfaces do not have implementation and only define method signatures, so there are no conflicts between multiple interfaces that a class implements.
Multiple implementations
Both Abstract Classes and Interfaces in Object Oriented Programming provide the mechanism for multiple implementations.
An abstract class can have both abstract and non-abstract/concrete methods. An abstract method is a method without an implementation that must be implemented by any concrete subclass. This allows for multiple implementations of the same functionality by different subclasses. Each subclass can provide its own implementation for the abstract methods inherited from the abstract class.
Interfaces, on the other hand, only contain abstract methods with no implementation. A class can implement one or more interfaces and provide a full implementation for all the abstract methods defined in the interfaces. This allows for multiple implementations of the same functionality by different classes that implement the same interface.
Both Abstract Classes and Interfaces provide the mechanism for multiple implementations, with Abstract Classes allowing for implementation of both abstract and concrete methods, and Interfaces allowing for implementation of only abstract methods.
When to use Abstract Class or Interface?
The choice between using an Abstract Class or an Interface in Object Oriented Programming depends on the specific requirements of the design.
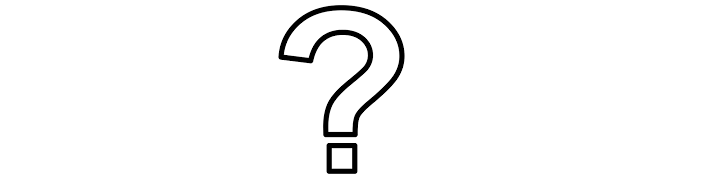
Abstract Classes are used when there is a common behavior that can be shared across multiple subclasses, and when some of the implementation can be shared while still allowing for subclass-specific implementation. Abstract Classes allow for inheritance of both abstract and concrete methods, and can provide a default implementation for some of the methods.
Interfaces, on the other hand, are used when there is a common behavior that needs to be implemented by multiple classes, but with no implementation shared between the classes. Interfaces only contain abstract methods, and provide a way for multiple classes to implement the same behavior, without creating a class hierarchy.
In summary, Abstract Classes are used when there is a common behavior that can be shared across multiple subclasses with some implementation shared, while Interfaces are used when there is a common behavior that needs to be implemented by multiple classes without any shared implementation.
- Java Interview Questions-Core Faq - 1
- Java Interview Questions-Core Faq - 2
- Java Interview Questions-Core Faq - 3
- Features of Java Programming Language (2024)
- Difference between Java and JavaScript?
- What is the difference between JDK and JRE?
- What gives Java its 'write once and run anywhere' nature?
- What is JVM and is it platform independent?
- What is Just-In-Time (JIT) compiler?
- What is the garbage collector in Java?
- What is NullPointerException in Java
- Difference between Stack and Heap memory in Java
- How to set the maximum memory usage for JVM?
- What is numeric promotion?
- Generics in Java
- Static keyword in Java
- What are final variables in Java?
- How Do Annotations Work in Java?
- How do I use the ternary operator in Java?
- What is instanceof keyword in Java?
- How ClassLoader Works in Java?
- What are fail-safe and fail-fast Iterators in Java
- What are method references in Java?
- "Cannot Find Symbol" compile error
- Difference between system.gc() and runtime.gc()
- How to convert TimeStamp to Date in Java?
- Does garbage collection guarantee that a program will not run out of memory?
- How setting an Object to null help Garbage Collection?
- How do objects become eligible for garbage collection?
- How to calculate date difference in Java
- Difference between Path and Classpath in Java
- Is Java "pass-by-reference" or "pass-by-value"?
- Difference between static and nonstatic methods java
- Why Java does not support pointers?
- What is a package in Java?
- What are wrapper classes in Java?
- What is singleton class in Java?
- Difference between Java Local Variable, Instance Variable and a Class Variable?
- Can a top level class be private or protected in Java
- Are Polymorphism , Overloading and Overriding similar concepts?
- Locking Mechanism in Java
- Why Multiple Inheritance is Not Supported in Java
- Why Java is not a pure Object Oriented language?
- Static class in Java
- Why do I need to override the equals and hashCode methods in Java?
- Why does Java not support operator overloading?
- Anonymous Classes in Java
- Static Vs Dynamic class loading in Java
- Why am I getting a NoClassDefFoundError in Java?
- How to Generate Random Number in Java
- What's the meaning of System.out.println in Java?
- What is the purpose of Runtime and System class in Java?
- The finally Block in Java
- Difference between final, finally and finalize
- What is try-with-resources in java?
- What is a stacktrace?
- Why String is immutable in Java ?
- What are different ways to create a string object in Java?
- Difference between String and StringBuffer/StringBuilder in Java
- Difference between creating String as new() and literal | Java
- How do I convert String to Date object in Java?
- How do I create a Java string from the contents of a file?
- What actually causes a StackOverflow error in Java?
- Why is char[] preferred over String for storage of password in Java
- What is I/O Filter and how do I use it in Java?
- Serialization and Deserialization in Java
- Understanding transient variables in Java
- What is Externalizable in Java?
- What is the purpose of serialization/deserialization in Java?
- What is the Difference between byte stream and Character streams
- How to append text to an existing file in Java
- How to convert InputStream object to a String in Java
- What is the difference between Reader and InputStream in Java
- Introduction to Java threads
- Synchronization in Java
- Static synchronization Vs non static synchronization in Java
- Deadlock in Java with Examples
- What is Daemon thread in Java
- Implement Runnable vs Extend Thread in Java
- What is the volatile keyword in Java
- What are the basic interfaces of Java Collections Framework
- Difference between ArrayList and Vector | Java
- What is the difference between ArrayList and LinkedList?
- What is the difference between List and Set in Java
- Difference between HashSet and HashMap in Java
- Difference between HashMap and Hashtable in Java?
- How does the hashCode() method of java works?
- Difference between capacity() and size() of Vector in Java
- What is a Java ClassNotFoundException?
- How to fix java.lang.UnsupportedClassVersionError