How to convert String to Date in Java
The SimpleDateFormat class in Java, which belongs to the java.text package, is a powerful tool for formatting and parsing dates according to specified patterns. It provides a straightforward and flexible way to handle date and time values in various formats.
Formatting Dates
One of the key features of SimpleDateFormat is its ability to format dates. You can specify a pattern that defines how the date should be displayed. The pattern consists of a combination of specific characters that represent different elements of a date, such as the year, month, day, hour, minute, and second. For example, the pattern "yyyy-MM-dd" represents a date in the format "year-month-day", such as "2023-06-29".
Here are some common pattern characters used in SimpleDateFormat:
- "y" represents the year.
- "M" represents the month.
- "d" represents the day.
- "H" represents the hour in 24-hour format.
- "h" represents the hour in 12-hour format.
- "m" represents the minute.
- "s" represents the second.
Parsing Dates
In addition to formatting, SimpleDateFormat also allows you to parse dates. Parsing refers to the process of converting a date string into a Date object, which can be used for further manipulation or calculations. You specify a pattern that matches the structure of the date string you want to parse, and SimpleDateFormat converts it into a Date object.
String to Date conversion
ExampleHere's an extract of all available format patterns from the javadoc:
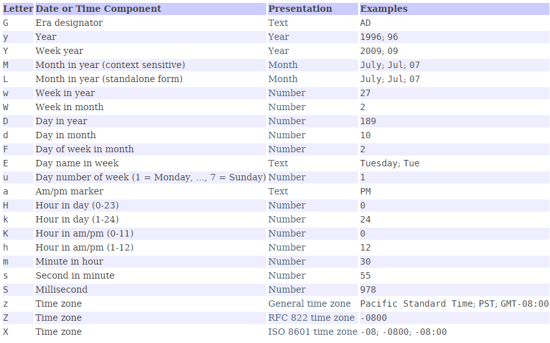
Convert different types of string to Date in java
Custom Patterns
One of the strengths of SimpleDateFormat is its flexibility to define custom patterns. You can create patterns that suit your specific requirements and match the desired date format. For instance, if you want to display the date as "June 29, 2023," you can create a custom pattern like "MMMM dd, yyyy".
Error Handling
When working with SimpleDateFormat, it's crucial to handle potential exceptions. ParseException is a checked exception that can be thrown when parsing a date string that doesn't match the specified pattern. It's important to handle this exception to manage invalid or unexpected date formats.
Thread Safety
It's worth noting that SimpleDateFormat is not thread-safe. If you plan to use SimpleDateFormat concurrently in a multi-threaded environment, you should either synchronize access to the SimpleDateFormat instance or consider using alternative thread-safe date formatting libraries like java.time.format.DateTimeFormatter introduced in Java 8.
Conclusion
SimpleDateFormat provides a convenient way to format and parse dates in Java. It allows you to control the display and interpretation of date and time values by defining patterns that align with your desired format.
- Java Interview Questions-Core Faq - 1
- Java Interview Questions-Core Faq - 2
- Java Interview Questions-Core Faq - 3
- Features of Java Programming Language (2024)
- Difference between Java and JavaScript?
- What is the difference between JDK and JRE?
- What gives Java its 'write once and run anywhere' nature?
- What is JVM and is it platform independent?
- What is Just-In-Time (JIT) compiler?
- What is the garbage collector in Java?
- What is NullPointerException in Java
- Difference between Stack and Heap memory in Java
- How to set the maximum memory usage for JVM?
- What is numeric promotion?
- Generics in Java
- Static keyword in Java
- What are final variables in Java?
- How Do Annotations Work in Java?
- How do I use the ternary operator in Java?
- What is instanceof keyword in Java?
- How ClassLoader Works in Java?
- What are fail-safe and fail-fast Iterators in Java
- What are method references in Java?
- "Cannot Find Symbol" compile error
- Difference between system.gc() and runtime.gc()
- How to convert TimeStamp to Date in Java?
- Does garbage collection guarantee that a program will not run out of memory?
- How setting an Object to null help Garbage Collection?
- How do objects become eligible for garbage collection?
- How to calculate date difference in Java
- Difference between Path and Classpath in Java
- Is Java "pass-by-reference" or "pass-by-value"?
- Difference between static and nonstatic methods java
- Why Java does not support pointers?
- What is a package in Java?
- What are wrapper classes in Java?
- What is singleton class in Java?
- Difference between Java Local Variable, Instance Variable and a Class Variable?
- Can a top level class be private or protected in Java
- Are Polymorphism , Overloading and Overriding similar concepts?
- Locking Mechanism in Java
- Why Multiple Inheritance is Not Supported in Java
- Why Java is not a pure Object Oriented language?
- Static class in Java
- Difference between Abstract class and Interface in Java
- Why do I need to override the equals and hashCode methods in Java?
- Why does Java not support operator overloading?
- Anonymous Classes in Java
- Static Vs Dynamic class loading in Java
- Why am I getting a NoClassDefFoundError in Java?
- How to Generate Random Number in Java
- What's the meaning of System.out.println in Java?
- What is the purpose of Runtime and System class in Java?
- The finally Block in Java
- Difference between final, finally and finalize
- What is try-with-resources in java?
- What is a stacktrace?
- Why String is immutable in Java ?
- What are different ways to create a string object in Java?
- Difference between String and StringBuffer/StringBuilder in Java
- Difference between creating String as new() and literal | Java
- How do I create a Java string from the contents of a file?
- What actually causes a StackOverflow error in Java?
- Why is char[] preferred over String for storage of password in Java
- What is I/O Filter and how do I use it in Java?
- Serialization and Deserialization in Java
- Understanding transient variables in Java
- What is Externalizable in Java?
- What is the purpose of serialization/deserialization in Java?
- What is the Difference between byte stream and Character streams
- How to append text to an existing file in Java
- How to convert InputStream object to a String in Java
- What is the difference between Reader and InputStream in Java
- Introduction to Java threads
- Synchronization in Java
- Static synchronization Vs non static synchronization in Java
- Deadlock in Java with Examples
- What is Daemon thread in Java
- Implement Runnable vs Extend Thread in Java
- What is the volatile keyword in Java
- What are the basic interfaces of Java Collections Framework
- Difference between ArrayList and Vector | Java
- What is the difference between ArrayList and LinkedList?
- What is the difference between List and Set in Java
- Difference between HashSet and HashMap in Java
- Difference between HashMap and Hashtable in Java?
- How does the hashCode() method of java works?
- Difference between capacity() and size() of Vector in Java
- What is a Java ClassNotFoundException?
- How to fix java.lang.UnsupportedClassVersionError