Difference between List and Set in Java
Both List and Set are interfaces that are part of the Java Collections Framework. However, they have different characteristics and purposes. Here's an explanation of the differences between List and Set:
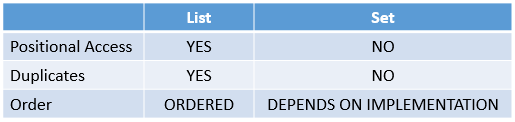
Definition and Purpose
- List: A List is an ordered collection that allows duplicate elements. It maintains the insertion order, meaning the elements are stored in the order they were added. Lists are used when the order of elements matters, and duplicates are allowed.
- Set: A Set is an unordered collection that does not allow duplicate elements. It does not maintain any specific order of elements. Sets are used when uniqueness of elements is important, and the order is not relevant.
Duplicate Elements
- List: Lists allow duplicate elements. You can have multiple elements with the same value in a List.
- Set: Sets do not allow duplicate elements. If you attempt to add an element that already exists in the Set, it will be ignored, and the Set will remain unchanged.
Order
- List: Lists maintain the order of elements. The order in which elements are added is preserved, and you can access elements by their index.
- Set: Sets do not maintain any specific order of elements. The order of elements in a Set can be arbitrary, and there is no index-based access to elements.
Key Operations
- List: Lists provide operations such as adding elements at a specific index, removing elements by index, retrieving elements by index, and iterating over elements in a predictable order.
- Set: Sets provide operations for adding elements, removing elements, checking for the existence of an element, and iterating over elements. The focus is on checking membership and uniqueness rather than index-based access.
Implementations
- List: Common implementations of List interface include ArrayList, LinkedList, and Vector.
- Set: Common implementations of Set interface include HashSet, LinkedHashSet, and TreeSet.
When to use
A List is a carefully arranged sequence of elements, where the order of insertion is strictly maintained. On the other hand, a Set represents a collection of distinct elements, which, unlike a List, lacks any particular ordering. Hence, when it comes to data storage, Lists serve as an excellent choice for preserving the original order of non-unique objects, while Sets shine in their ability to house exclusively unique objects, albeit in an arbitrary arrangement.
Developers can rely on its inherent ability to maintain a precise order of elements. This feature proves invaluable when there is a requirement to retain the original sequence in which objects were added. Lists are especially suitable for scenarios where duplicate entries are permissible and need to be captured without altering their relative positions. For example, in a task management system, a List can preserve the chronological order of tasks as they are added, allowing for easy retrieval and tracking based on their insertion timestamps.
A Set operates on the principle of distinctness, ensuring that each element exists in the collection only once. By using a Set, programmers can guarantee uniqueness, as duplicate elements are automatically eliminated. This characteristic is particularly useful in situations where redundancy must be avoided, such as when storing user credentials or a collection of unique identifiers. Although Sets do not enforce any specific order, they provide efficient membership checking, allowing developers to quickly determine if an element is present within the collection.
- Java Interview Questions-Core Faq - 1
- Java Interview Questions-Core Faq - 2
- Java Interview Questions-Core Faq - 3
- Features of Java Programming Language (2024)
- Difference between Java and JavaScript?
- What is the difference between JDK and JRE?
- What gives Java its 'write once and run anywhere' nature?
- What is JVM and is it platform independent?
- What is Just-In-Time (JIT) compiler?
- What is the garbage collector in Java?
- What is NullPointerException in Java
- Difference between Stack and Heap memory in Java
- How to set the maximum memory usage for JVM?
- What is numeric promotion?
- Generics in Java
- Static keyword in Java
- What are final variables in Java?
- How Do Annotations Work in Java?
- How do I use the ternary operator in Java?
- What is instanceof keyword in Java?
- How ClassLoader Works in Java?
- What are fail-safe and fail-fast Iterators in Java
- What are method references in Java?
- "Cannot Find Symbol" compile error
- Difference between system.gc() and runtime.gc()
- How to convert TimeStamp to Date in Java?
- Does garbage collection guarantee that a program will not run out of memory?
- How setting an Object to null help Garbage Collection?
- How do objects become eligible for garbage collection?
- How to calculate date difference in Java
- Difference between Path and Classpath in Java
- Is Java "pass-by-reference" or "pass-by-value"?
- Difference between static and nonstatic methods java
- Why Java does not support pointers?
- What is a package in Java?
- What are wrapper classes in Java?
- What is singleton class in Java?
- Difference between Java Local Variable, Instance Variable and a Class Variable?
- Can a top level class be private or protected in Java
- Are Polymorphism , Overloading and Overriding similar concepts?
- Locking Mechanism in Java
- Why Multiple Inheritance is Not Supported in Java
- Why Java is not a pure Object Oriented language?
- Static class in Java
- Difference between Abstract class and Interface in Java
- Why do I need to override the equals and hashCode methods in Java?
- Why does Java not support operator overloading?
- Anonymous Classes in Java
- Static Vs Dynamic class loading in Java
- Why am I getting a NoClassDefFoundError in Java?
- How to Generate Random Number in Java
- What's the meaning of System.out.println in Java?
- What is the purpose of Runtime and System class in Java?
- The finally Block in Java
- Difference between final, finally and finalize
- What is try-with-resources in java?
- What is a stacktrace?
- Why String is immutable in Java ?
- What are different ways to create a string object in Java?
- Difference between String and StringBuffer/StringBuilder in Java
- Difference between creating String as new() and literal | Java
- How do I convert String to Date object in Java?
- How do I create a Java string from the contents of a file?
- What actually causes a StackOverflow error in Java?
- Why is char[] preferred over String for storage of password in Java
- What is I/O Filter and how do I use it in Java?
- Serialization and Deserialization in Java
- Understanding transient variables in Java
- What is Externalizable in Java?
- What is the purpose of serialization/deserialization in Java?
- What is the Difference between byte stream and Character streams
- How to append text to an existing file in Java
- How to convert InputStream object to a String in Java
- What is the difference between Reader and InputStream in Java
- Introduction to Java threads
- Synchronization in Java
- Static synchronization Vs non static synchronization in Java
- Deadlock in Java with Examples
- What is Daemon thread in Java
- Implement Runnable vs Extend Thread in Java
- What is the volatile keyword in Java
- What are the basic interfaces of Java Collections Framework
- Difference between ArrayList and Vector | Java
- What is the difference between ArrayList and LinkedList?
- Difference between HashSet and HashMap in Java
- Difference between HashMap and Hashtable in Java?
- How does the hashCode() method of java works?
- Difference between capacity() and size() of Vector in Java
- What is a Java ClassNotFoundException?
- How to fix java.lang.UnsupportedClassVersionError