How do I resolve ClassNotFoundException?
As the nomenclature implies, a ClassNotFoundException in Java arises when the Java Virtual Machine (JVM) endeavors to load a specific class but fails to locate the requested class within the specified classpath. This predicament often arises due to a broken classpath, a prevalent issue in the Java area. For neophyte Java programmers, this can be a perplexing challenge. It is essential to note that ClassNotFoundException is a checked exception, mandating either its capture within a catch block or propagation to the caller through the throws clause, in order to maintain proper exception handling and ensure the program's stability.
Java ClassNotFoundException sample
One of the most frequent scenarios leading to a ClassNotFoundException is encountered when attempting to load JDBC drivers using Class.forName, yet inadvertently neglecting to include the corresponding JAR file in the classpath. This omission results in the Java Virtual Machine (JVM) being unable to find the specified driver class, leading to the ClassNotFoundException being thrown. To rectify this situation, it is imperative to ensure that the necessary JAR file containing the JDBC driver is appropriately added to the classpath, thereby facilitating the seamless loading of the desired class during runtime.
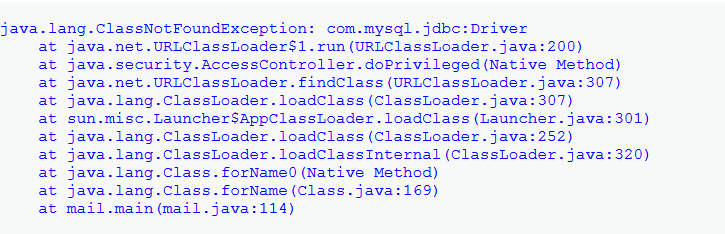
When ClassNotFoundException occurs in Java
Java ClassNotFoundException thrown when an application tries to load in a class through its string name using:
- The forName method in class Class.
- The findSystemClass method in class ClassLoader .
- The loadClass method in class ClassLoader.
Java ClassNotFoundException example
In the following example, there is no such class exist NoClassExist.java and try to attempt to load the class "NoClassExist".
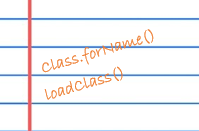
After the successful compilation of your Java code, the resulting output comprises .class files, which contain the bytecode interpreted by Java during program execution. However, instances of ClassNotFoundException may arise when attempting to load classes dynamically at runtime using methods like Class.forName() or loadClass(), but the requested classes cannot be located within the specified classpath. Another situation leading to this exception involves the presence of multiple class loaders, where one ClassLoader attempts to access a class that has already been loaded by another ClassLoader. This scenario may also trigger a ClassNotFoundException in Java. To address such occurrences, careful management of the classpath and handling of class loading mechanisms is crucial for seamless program execution.
Exception Hierarchy
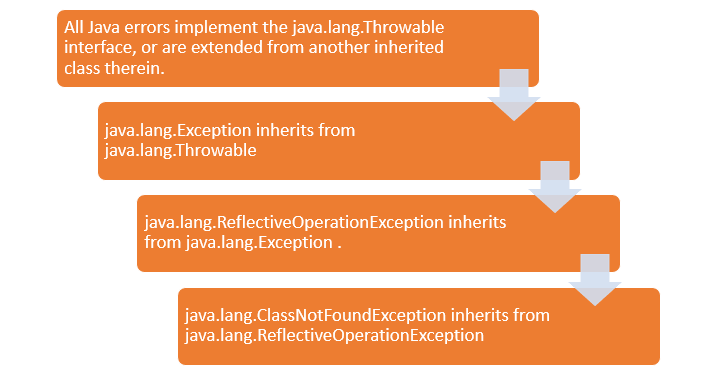
How to fix the ClassNotFoundException
- Review properly the java.lang.ClassNotFoundException stack trace which Java class was not loaded properly at runtime.
- Verify the name of the requested class is correct and the specified .jar file exists in your classpath. If not, you must explicitly add it to your application’s classpath.
- If it's present in your classpath then there is high chance that your classpath is getting overridden or application is using classpath specified in jar file or start-up script and to fix that you need to find the exact classpath used by your application.
- In case the exception is caused by a third party class, you must identify the class that throws the exception and then, add the missing .jar files in your classpath.
Java Classpath and ClassNotFoundException
- Java classpath is a list of locations to load classes from. These locations can either be directories, or jar files. For directories, the Java Virtual Machine will follow an expected pattern for loading a class.
- For example, if you have the directory C:/java/classes in your classpath, and you attempt to load a class com.myproject.myprog, it will look under the classes directory for a directory called com, then under that a directory called myproject, and finally it will look for a file called myprog.class in that directory.
- In case of jar files, it will search the jar file for that class. A jar file is a zipped collection of directories like the above. If you unzip a jar file, you'll get a bunch of directories and class files following the pattern above.
- So the Java Virtual Machine traverses a classpath from start to finish looking for the definition of the class when it attempts to load the class definition. For example, in the classpath :
- The Java Virtual Machine will attempt to look in the directory classes first, then in abc.jar and finally in xyz.jar.
Conclusion
When a ClassNotFoundException is encountered, it indicates that the Java Virtual Machine (JVM) has diligently searched through the entirety of the specified classpath, yet the targeted class remains elusive. In such situations, the primary and sole recourse is to carefully scrutinize the classpath configuration. Ensuring that the classpath is accurately set up to include the required class and its dependencies is imperative to resolving this exception. Thoroughly verifying the classpath configuration will pave the way for the successful location and loading of the desired class during runtime execution.
- Java Interview Questions-Core Faq - 1
- Java Interview Questions-Core Faq - 2
- Java Interview Questions-Core Faq - 3
- Features of Java Programming Language (2024)
- Difference between Java and JavaScript?
- What is the difference between JDK and JRE?
- What gives Java its 'write once and run anywhere' nature?
- What is JVM and is it platform independent?
- What is Just-In-Time (JIT) compiler?
- What is the garbage collector in Java?
- What is NullPointerException in Java
- Difference between Stack and Heap memory in Java
- How to set the maximum memory usage for JVM?
- What is numeric promotion?
- Generics in Java
- Static keyword in Java
- What are final variables in Java?
- How Do Annotations Work in Java?
- How do I use the ternary operator in Java?
- What is instanceof keyword in Java?
- How ClassLoader Works in Java?
- What are fail-safe and fail-fast Iterators in Java
- What are method references in Java?
- "Cannot Find Symbol" compile error
- Difference between system.gc() and runtime.gc()
- How to convert TimeStamp to Date in Java?
- Does garbage collection guarantee that a program will not run out of memory?
- How setting an Object to null help Garbage Collection?
- How do objects become eligible for garbage collection?
- How to calculate date difference in Java
- Difference between Path and Classpath in Java
- Is Java "pass-by-reference" or "pass-by-value"?
- Difference between static and nonstatic methods java
- Why Java does not support pointers?
- What is a package in Java?
- What are wrapper classes in Java?
- What is singleton class in Java?
- Difference between Java Local Variable, Instance Variable and a Class Variable?
- Can a top level class be private or protected in Java
- Are Polymorphism , Overloading and Overriding similar concepts?
- Locking Mechanism in Java
- Why Multiple Inheritance is Not Supported in Java
- Why Java is not a pure Object Oriented language?
- Static class in Java
- Difference between Abstract class and Interface in Java
- Why do I need to override the equals and hashCode methods in Java?
- Why does Java not support operator overloading?
- Anonymous Classes in Java
- Static Vs Dynamic class loading in Java
- Why am I getting a NoClassDefFoundError in Java?
- How to Generate Random Number in Java
- What's the meaning of System.out.println in Java?
- What is the purpose of Runtime and System class in Java?
- The finally Block in Java
- Difference between final, finally and finalize
- What is try-with-resources in java?
- What is a stacktrace?
- Why String is immutable in Java ?
- What are different ways to create a string object in Java?
- Difference between String and StringBuffer/StringBuilder in Java
- Difference between creating String as new() and literal | Java
- How do I convert String to Date object in Java?
- How do I create a Java string from the contents of a file?
- What actually causes a StackOverflow error in Java?
- Why is char[] preferred over String for storage of password in Java
- What is I/O Filter and how do I use it in Java?
- Serialization and Deserialization in Java
- Understanding transient variables in Java
- What is Externalizable in Java?
- What is the purpose of serialization/deserialization in Java?
- What is the Difference between byte stream and Character streams
- How to append text to an existing file in Java
- How to convert InputStream object to a String in Java
- What is the difference between Reader and InputStream in Java
- Introduction to Java threads
- Synchronization in Java
- Static synchronization Vs non static synchronization in Java
- Deadlock in Java with Examples
- What is Daemon thread in Java
- Implement Runnable vs Extend Thread in Java
- What is the volatile keyword in Java
- What are the basic interfaces of Java Collections Framework
- Difference between ArrayList and Vector | Java
- What is the difference between ArrayList and LinkedList?
- What is the difference between List and Set in Java
- Difference between HashSet and HashMap in Java
- Difference between HashMap and Hashtable in Java?
- How does the hashCode() method of java works?
- Difference between capacity() and size() of Vector in Java
- How to fix java.lang.UnsupportedClassVersionError